How to add HexaDecimal Color Codes in Android Jetpack Compose
Hex color codes are one of the most used color formats while developing mobile apps and websites. This is a short Android tutorial on how to use hex color codes in Jetpack Compose.
There’s no straightforward way to give hex color codes in Jetpack Compose. Still, you can use them by just appending 0xFF to the start of the hex code. For example, if the hex code is #27AE60 then just use 0xFF27AE60 as given in the following code snippet.
@Composable
fun Example() {
Box(modifier = Modifier.width(100.dp).height(100.dp).background(color = Color(0xFF27AE60)))
}
Following is the complete code for your reference.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.width
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
Example()
}
}
}
}
}
}
@Composable
fun Example() {
Box(modifier = Modifier.width(100.dp).height(100.dp).background(color = Color(0xFF27AE60)))
}
You will get the output as given below.
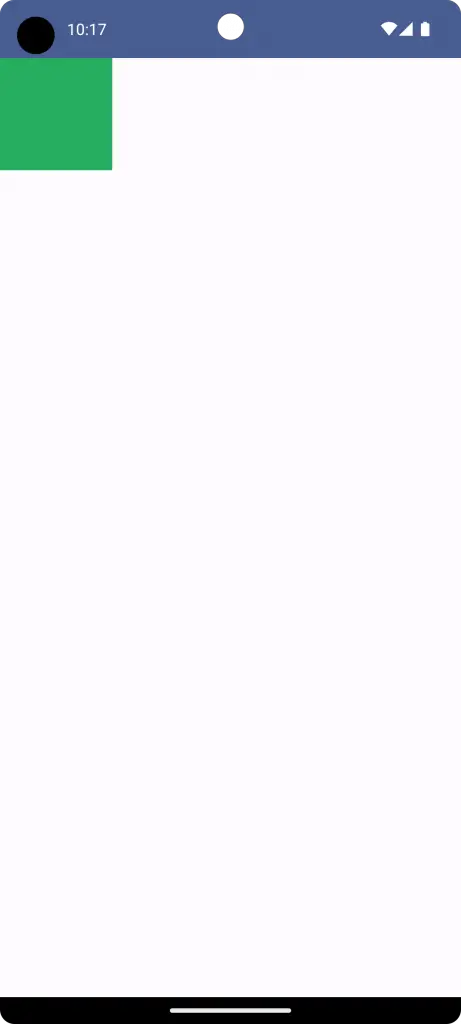
That’s how you add hex color codes in Jetpack Compose easily.