How to add Text with Border in Android Jetpack Compose
Borders can make UI elements beautiful. Let’s learn how to add Borders to text in Jetpack Compose.
Text composable has many useful parameters. In this case, the Modifier comes in handy. You can show borders using BorderStroke. See the code snippet given below.
Jetpack Compose Text with Border
@Composable
fun TextExample() {
Text( modifier = Modifier.padding(20.dp).border(BorderStroke(2.dp, Color.Red)),
text = "This is a sample text. This is an example of adding border to text.",
fontSize = 24.sp)
}
The output is given below.
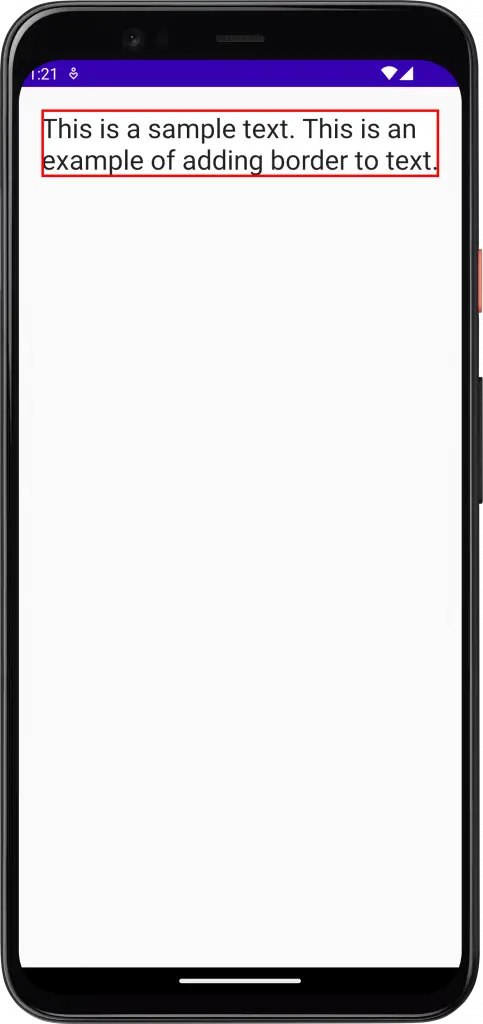
Complete Code
Following is the complete code.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.BorderStroke
import androidx.compose.foundation.border
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextExample()
}
}
}
}
}
}
@Composable
fun TextExample() {
Text( modifier = Modifier.padding(20.dp).border(BorderStroke(2.dp, Color.Red)),
text = "This is a sample text. This is an example of adding border to text.",
fontSize = 24.sp)
}
If you want more control over the border then it is better to use UI elements such as Card.
One Comment