How to Change Jetpack Compose Switch Thumb Color
Jetpack Compose, Android’s modern UI toolkit, empowers developers to create highly customizable interfaces with ease. One aspect of this customization is altering the thumb color of a Switch
component.
This blog post delves into how to change the thumb color of a switch in Jetpack Compose, adding a touch of personalization and coherence to your app’s UI.
In Jetpack Compose, a Switch
is an interactive UI component used for toggling between two states. The thumb is the circular part that slides from one end to the other, indicating the current state. Customizing its color can enhance the visual appeal and make the UI more intuitive.
Customize the Thumb Color
The Basics of Switch Customization
Jetpack Compose makes it straightforward to customize the switch’s thumb color. This is done through the SwitchDefaults
object, which provides various options for customizing a switch’s appearance.
Implement Custom Thumb Color
To change the thumb color, you use the colors
parameter of the Switch
composable. This parameter accepts a SwitchColors
object, which allows you to define the thumb color for different states of the switch.
Example:
@Composable
fun MySwitch() {
var isChecked by remember { mutableStateOf(true) }
Switch(
checked = isChecked,
onCheckedChange = { isChecked = it },
colors = SwitchDefaults.colors(
checkedThumbColor = Color.Red,
uncheckedThumbColor = Color.Gray
)
)
}
In this example, the thumb color is red when the switch is ON and gray when OFF.
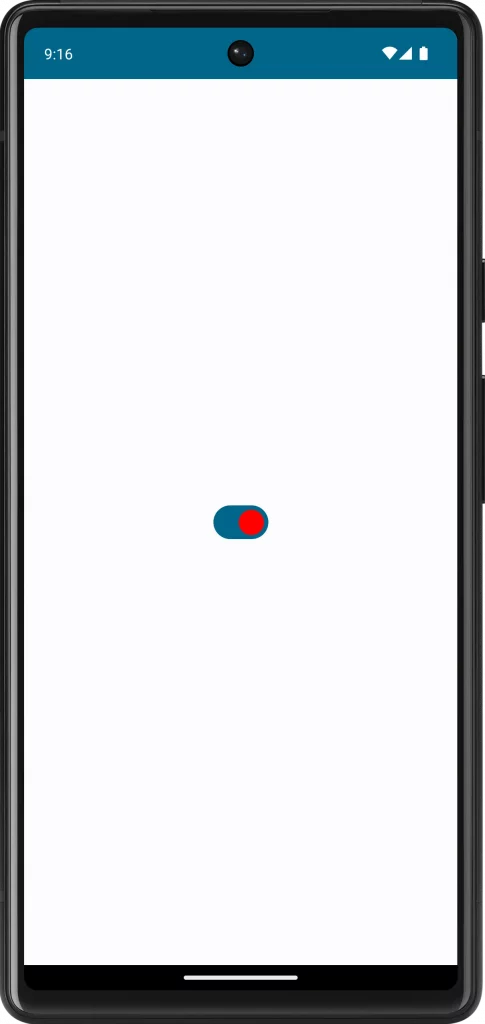
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Switch
import androidx.compose.material3.SwitchDefaults
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
MySwitch()
}
}
}
}
}
@Composable
fun MySwitch() {
var isChecked by remember { mutableStateOf(true) }
Switch(
checked = isChecked,
onCheckedChange = { isChecked = it },
colors = SwitchDefaults.colors(
checkedThumbColor = Color.Red,
uncheckedThumbColor = Color.Gray
)
)
}
Customizing the thumb color of a switch in Jetpack Compose is an effective way to add a personalized touch to your app’s UI. By selecting appropriate colors and considering consistency and accessibility, you can enhance the visual appeal and user experience of your Android application.