How to Add Space Between Row Items in Jetpack Compose
In Jetpack Compose, designing an intuitive and visually appealing UI is a key aspect of Android development. A common challenge is spacing elements within a Row
.
This blog post focuses on different methods to efficiently add space between row items in Jetpack Compose.
A Row
in Jetpack Compose arranges its children horizontally. By default, these children are placed directly next to each other without any space. Managing this space is important for creating a visually appealing and user-friendly interface.
Spacing Techniques in Jetpack Compose Rows
Let’s explore various ways to manage spacing between elements in a Row
.
Use Arrangement.SpaceBetween
This approach distributes the available space evenly between the children in a Row
. It places the first item at the start and the last item at the end, spacing out the remaining items evenly in between.
Example:
Row(horizontalArrangement = Arrangement.SpaceBetween) {
Text("Item 1")
Text("Item 2")
Text("Item 3")
}
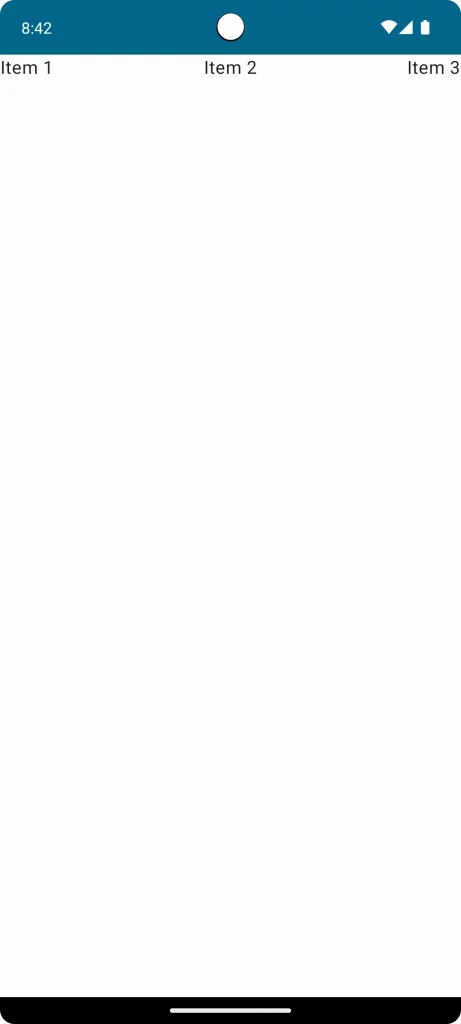
Use Spacer
Spacer
is an invisible composable that can be assigned a specific width, creating a fixed space between elements.
Example:
Row {
Text("Item 1")
Spacer(modifier = Modifier.width(16.dp))
Text("Item 2")
}
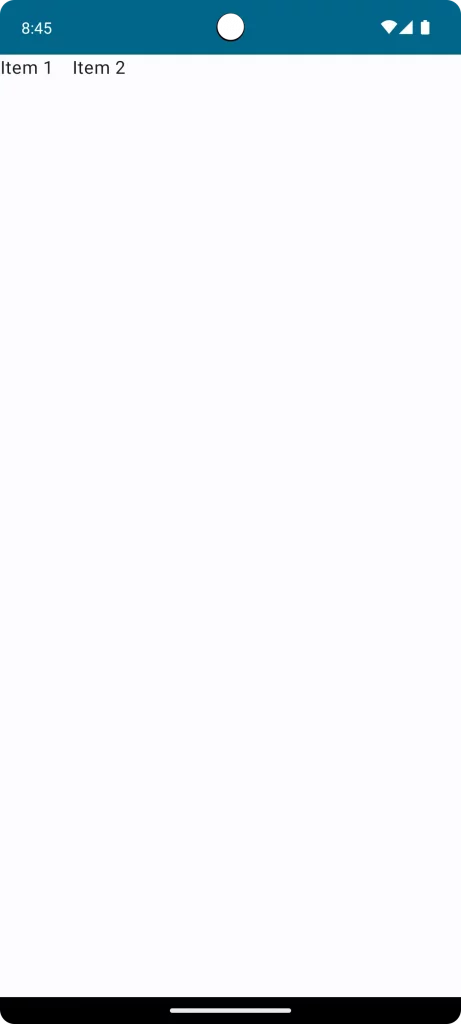
Use horizontalArrangement with spacedBy
Arrangement.spacedBy
allows for predefined space between each item in a Row
.
Example:
Row(horizontalArrangement = Arrangement.spacedBy(16.dp)) {
Text("Item 1")
Text("Item 2")
}
Use Modifier.padding
Individual padding to children within the row also creates space. This method offers more control over each element.
Example:
Row {
Text("Item 1", modifier = Modifier.padding(end = 16.dp))
Text("Item 2")
}
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Row
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Example()
}
}
}
}
}
@Composable
fun Example() {
Row {
Text("Item 1", modifier = Modifier.padding(end = 16.dp))
Text("Item 2")
}
}
Effectively spacing items in a Row
in Jetpack Compose is important for a clean and user-friendly UI. Whether you choose Arrangement.SpaceBetween
, Spacer
, Arrangement.spacedBy
, or padding, each method offers unique advantages. Opt for the one that aligns with your layout requirements and design vision.