How to Create a DropDown Menu in Android Jetpack Compose
Dropdown menus are a common UI component in many applications. They help users make selections from a list of options. In this blog post, we’ll explore how to implement a dropdown menu using Jetpack Compose, Google’s modern Android UI toolkit.
The DropDown Menu Code Example
Here’s a simple example code snippet that demonstrates how to create a drop-down menu in Jetpack Compose.
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun DropDownExample() {
val options = listOf("Option 1", "Option 2", "Option 3")
var expanded by remember { mutableStateOf(false) }
var selectedOptionText by remember { mutableStateOf(options[0]) }
ExposedDropdownMenuBox(
expanded = expanded,
onExpandedChange = { expanded = !expanded },
) {
TextField(
modifier = Modifier.menuAnchor(),
readOnly = true,
value = selectedOptionText,
onValueChange = {},
label = { Text("Label") },
trailingIcon = { ExposedDropdownMenuDefaults.TrailingIcon(expanded = expanded) },
colors = ExposedDropdownMenuDefaults.textFieldColors(),
)
ExposedDropdownMenu(
expanded = expanded,
onDismissRequest = { expanded = false },
) {
options.forEach { selectionOption ->
DropdownMenuItem(
text = { Text(selectionOption) },
onClick = {
selectedOptionText = selectionOption
expanded = false
},
)
}
}
}
}
Code Explanation
Let’s break down the code to understand how each part works:
Declaring State Variables
options
is a list of string values that will populate the drop-down menu.expanded
keeps track of whether the drop-down menu is open or closed.selectedOptionText
stores the currently selected option from the menu.
ExposedDropdownMenuBox
- This Composable is a container for the drop-down menu. It takes the
expanded
andonExpandedChange
parameters.
TextField
- The
TextField
displays the selected option. It’s read-only and uses themenuAnchor
modifier for positioning. - It also has a trailing icon that changes based on the
expanded
state.
ExposedDropdownMenu and DropdownMenuItem
ExposedDropdownMenu
is the actual drop-down list.- Each
DropdownMenuItem
represents an option within the drop-down list.
Event Handling
- The
onExpandedChange
andonDismissRequest
events are used to toggle the visibility of the drop-down menu. onClick
withinDropdownMenuItem
is used to update the selected option.
Complete Code
To incorporate this drop-down menu into your Android project, simply add the DropDownExample()
function inside your main activity’s content like so:
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.DropdownMenuItem
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.ExposedDropdownMenuBox
import androidx.compose.material3.ExposedDropdownMenuDefaults
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TextField
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
DropDownExample()
}
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun DropDownExample() {
val options = listOf("Option 1", "Option 2", "Option 3")
var expanded by remember { mutableStateOf(false) }
var selectedOptionText by remember { mutableStateOf(options[0]) }
ExposedDropdownMenuBox(
expanded = expanded,
onExpandedChange = { expanded = !expanded },
) {
TextField(
modifier = Modifier.menuAnchor(),
readOnly = true,
value = selectedOptionText,
onValueChange = {},
label = { Text("Label") },
trailingIcon = { ExposedDropdownMenuDefaults.TrailingIcon(expanded = expanded) },
colors = ExposedDropdownMenuDefaults.textFieldColors(),
)
ExposedDropdownMenu(
expanded = expanded,
onDismissRequest = { expanded = false },
) {
options.forEach { selectionOption ->
DropdownMenuItem(
text = { Text(selectionOption) },
onClick = {
selectedOptionText = selectionOption
expanded = false
},
)
}
}
}
}
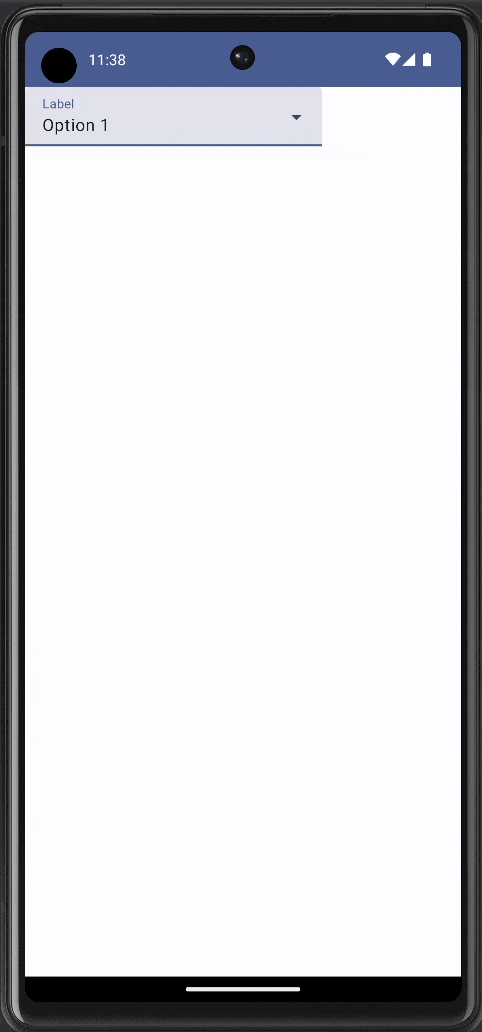
Creating a drop-down menu in Jetpack Compose is quite simple yet powerful. With just a few lines of code, you can implement a functional and good-looking drop-down menu in your Android application.