How to Create Custom Dialog in Android Jetpack Compose
Dialogs are an integral part of any mobile application. They are typically used to grab the user’s attention, provide additional information, or get user input. In Android Jetpack Compose, creating custom dialogs is not only simple but also fun, thanks to the power and flexibility of declarative UI patterns.
This blog post illustrates how to create and customize dialogs in Jetpack Compose using a hands-on example.
In Jetpack Compose, a dialog can be created using the Dialog composable function. This function provides a onDismissRequest callback that can be utilized to hide the dialog when the user clicks outside of the dialog. Here’s an example of a simple custom dialog.
@Composable
fun DialogExample() {
var showDialog by remember { mutableStateOf(false) }
Column {
Button(onClick = { showDialog = true }) {
Text(text = "Click to Show Dialog" )
}
}
if (showDialog) {
Dialog(onDismissRequest = {showDialog = false}) {
// Custom shape, background, and layout for the dialog
Surface(
shape = RoundedCornerShape(16.dp),
) {
Column(
modifier = Modifier.padding(16.dp).fillMaxWidth(),
horizontalAlignment = Alignment.CenterHorizontally
) {
Text("This is a custom dialog")
Button(
onClick = {showDialog = false},
modifier = Modifier.padding(top = 16.dp)
) {
Text("Close Dialog")
}
}
}
}
}
}
In the above example, we create a Button that when clicked, shows the dialog. The dialog content is wrapped with a Surface composable that allows us to easily style our dialog.
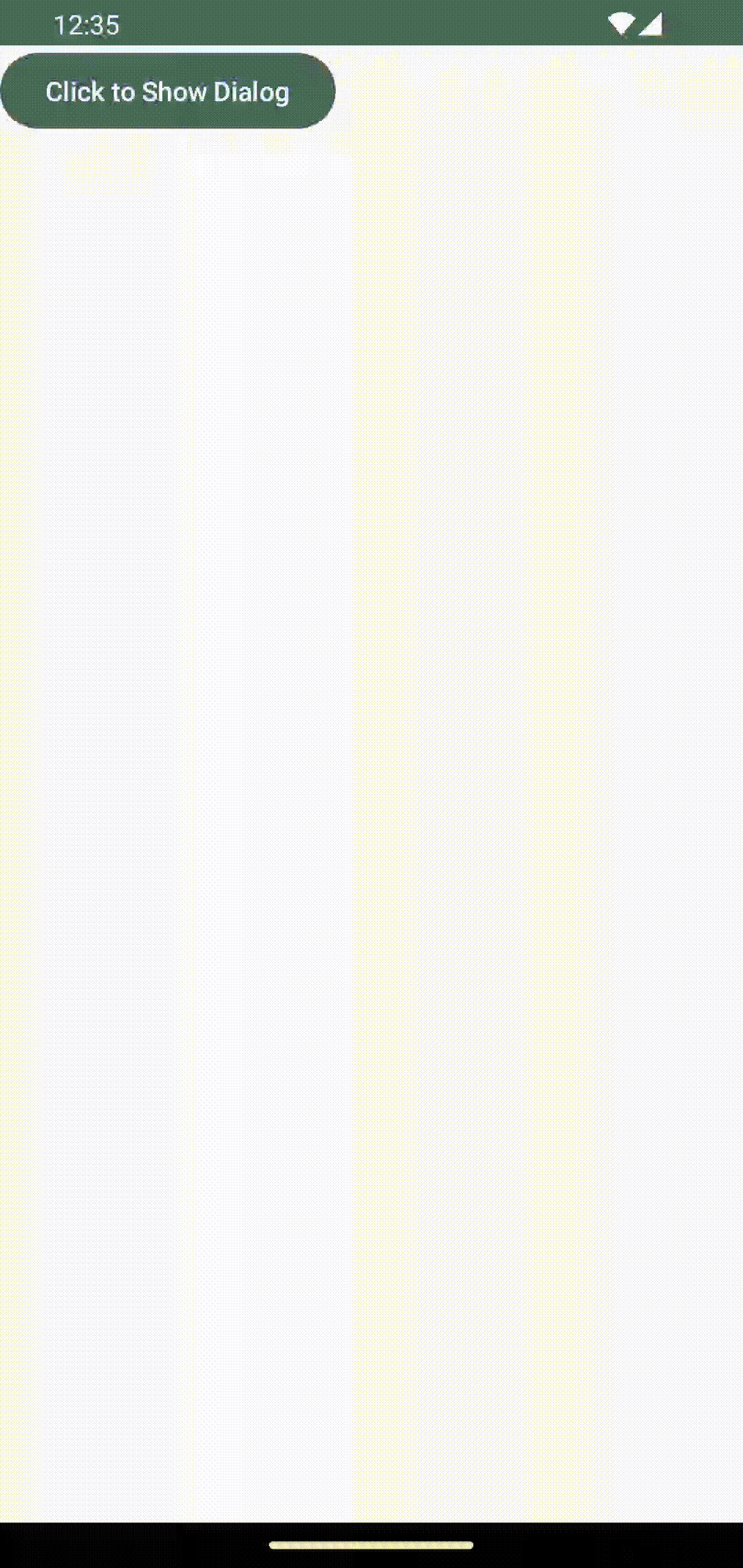
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.shape.RoundedCornerShape
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import androidx.compose.ui.window.Dialog
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
DialogExample()
}
}
}
}
}
@Composable
fun DialogExample() {
var showDialog by remember { mutableStateOf(false) }
Column {
Button(onClick = { showDialog = true }) {
Text(text = "Click to Show Dialog" )
}
}
if (showDialog) {
Dialog(onDismissRequest = {showDialog = false}) {
// Custom shape, background, and layout for the dialog
Surface(
shape = RoundedCornerShape(16.dp),
) {
Column(
modifier = Modifier.padding(16.dp).fillMaxWidth(),
horizontalAlignment = Alignment.CenterHorizontally
) {
Text("This is a custom dialog")
Button(
onClick = {showDialog = false},
modifier = Modifier.padding(top = 16.dp)
) {
Text("Close Dialog")
}
}
}
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
DialogExample()
}
}
Creating and customizing dialogs in Jetpack Compose is quite straightforward. With its powerful composable functions, you can easily build dialogs that are both aesthetically pleasing and functional.