How to Create Full Screen Dialog in Android Jetpack Compose
Dialogs are an important component of any mobile application. They are typically used to capture user input or provide crucial information to the user. Android Jetpack Compose, the modern UI toolkit for Android, simplifies the process of creating and managing dialogs.
In this blog post, we will demonstrate how to create a full screen dialog in Jetpack Compose.
Full Screen Dialog in Jetpack Compose
Creating a full screen dialog in Jetpack Compose is straightforward. Here is an example of how you can achieve this:
@Composable
fun FullScreenDialogExample() {
var showDialog by remember { mutableStateOf(false) }
Column {
Button(onClick = { showDialog = true }) {
Text(text = "Click to Show Full Screen Dialog" )
}
}
if (showDialog) {
Dialog(onDismissRequest = {showDialog = false}, properties = DialogProperties(usePlatformDefaultWidth = false)) {
// Custom layout for the dialog
Surface(
modifier = Modifier.fillMaxSize(),
shape = RoundedCornerShape(0.dp),
color = Color.Yellow
) {
Column(
modifier = Modifier.padding(16.dp).fillMaxWidth(),
horizontalAlignment = Alignment.CenterHorizontally
) {
Text("This is a full screen dialog")
Button(
onClick = {showDialog = false},
modifier = Modifier.padding(top = 16.dp)
) {
Text("Close Dialog")
}
}
}
}
}
}
The code above creates a composable function FullScreenDialogExample which when invoked, displays a button on the screen. On pressing the button, a full screen dialog is displayed.
The Dialog composable is used to display the full screen dialog. To make the dialog full screen, we set the usePlatformDefaultWidth property of DialogProperties to false, and provide Modifier.fillMaxSize() to the Surface composable. This fills the entire screen with our dialog.
In the Surface composable, we create a column that holds a Text and a Button composable. The Button composable has an onClick event that dismisses the dialog when clicked.
That’s it! You have now created a full screen dialog using Jetpack Compose.
As with everything in Jetpack Compose, the dialog will automatically recompose when the state changes, such as when the showDialog variable is updated.
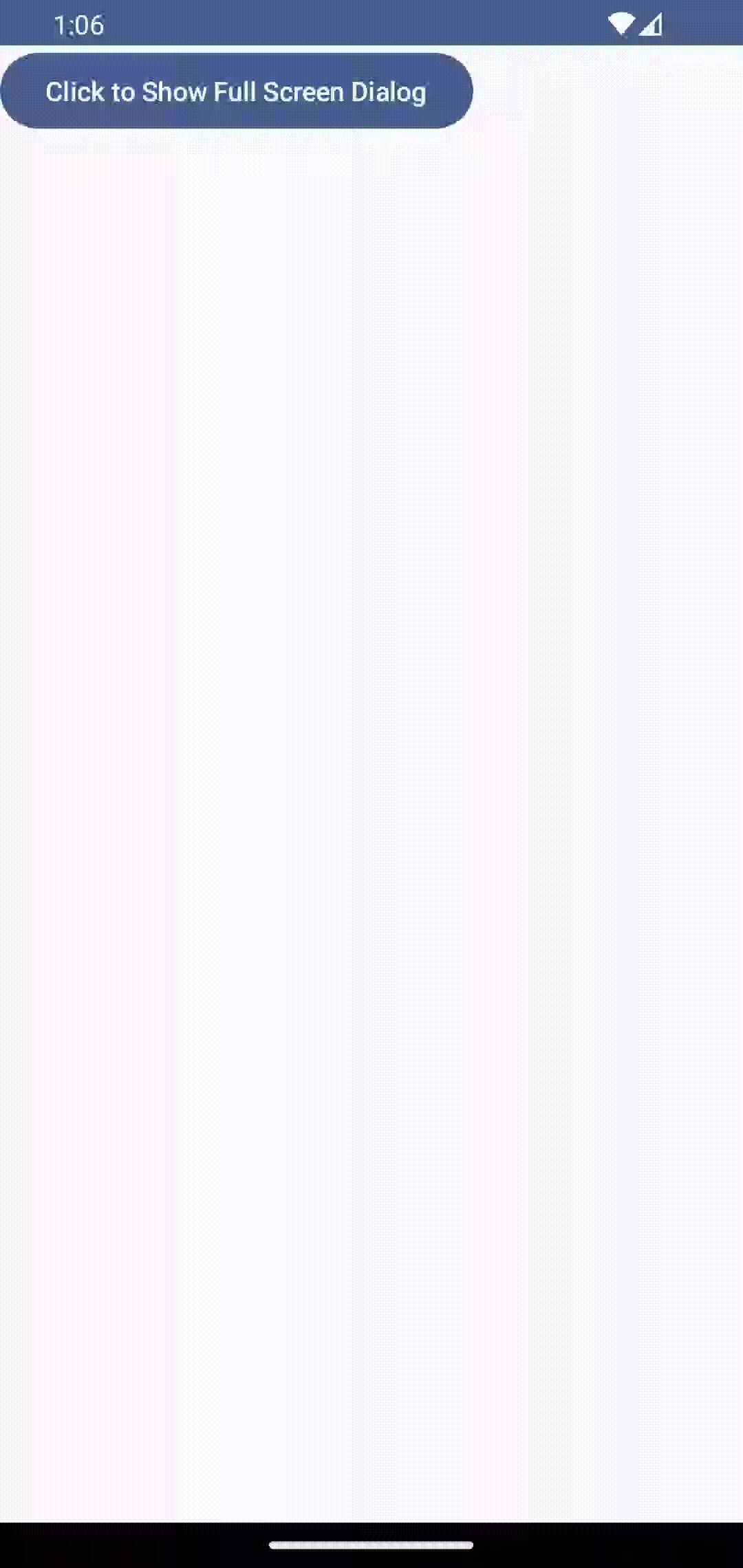
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.shape.RoundedCornerShape
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import androidx.compose.ui.window.Dialog
import androidx.compose.ui.window.DialogProperties
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
FullScreenDialogExample()
}
}
}
}
}
@Composable
fun FullScreenDialogExample() {
var showDialog by remember { mutableStateOf(false) }
Column {
Button(onClick = { showDialog = true }) {
Text(text = "Click to Show Full Screen Dialog" )
}
}
if (showDialog) {
Dialog(onDismissRequest = {showDialog = false}, properties = DialogProperties(usePlatformDefaultWidth = false)) {
// Custom layout for the dialog
Surface(
modifier = Modifier.fillMaxSize(),
shape = RoundedCornerShape(0.dp),
color = Color.Yellow
) {
Column(
modifier = Modifier.padding(16.dp).fillMaxWidth(),
horizontalAlignment = Alignment.CenterHorizontally
) {
Text("This is a full screen dialog")
Button(
onClick = {showDialog = false},
modifier = Modifier.padding(top = 16.dp)
) {
Text("Close Dialog")
}
}
}
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
FullScreenDialogExample()
}
}
Jetpack Compose provides a straightforward way to create full screen dialogs and other UI components in Android. It’s simple to handle state changes, making it much easier to build dynamic and interactive dialogs. As you have seen in this tutorial, you can create a full screen dialog with just a few lines of code.