Difference Between Surface and Box in Android Jetpack Compose
In Android’s Jetpack Compose, Surface and Box are two vital layout components that you will likely come across often. While they might appear similar at first glance, they serve different purposes and have distinct characteristics.
This blog post will delve into the differences between Surface and Box to help you understand when to use each in your application.
What is a Box?
Box is a layout component provided by Jetpack Compose that stacks its children on top of each other. It is often used when you want to layer multiple composables or if you want to align a single child to a particular position within it. For instance, you might want to place an icon at the top-right corner of an image; this can be easily achieved using a Box.
Here’s an example:
@Composable
fun Example() {
Column {
Box(modifier = Modifier.size(200.dp)) {
Image(
painter = painterResource(id = R.drawable.nature),
contentDescription = "Example Image",
modifier = Modifier.size(200.dp),
contentScale = ContentScale.Crop
)
Icon(
modifier = Modifier.align(Alignment.TopEnd),
imageVector = Icons.Default.Close,
contentDescription = "Home Icon"
)
}
}
}
In this example, the Icon is placed at the top-end of the Box, overlaying the Image.
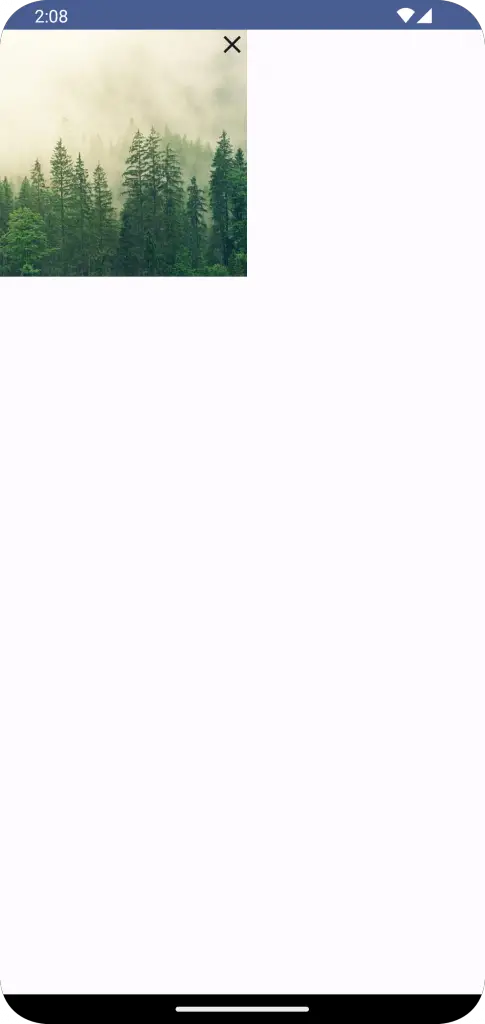
What is a Surface?
Surface is a layout component that provides a container for your content, applying Material Design styles such as elevation, shape, and color. Surface also respects the light/dark theme modes of your app, automatically adjusting its colors based on the current theme.
An example of using Surface is as follows:
@Composable
fun Example() {
Column {
Surface(
modifier = Modifier.size(300.dp).padding(20.dp),
color = MaterialTheme.colorScheme.primary,
shape = RoundedCornerShape(32.dp),
shadowElevation = 16.dp
) {
Text("Hello Jetpack Compose", Modifier.padding(16.dp))
}
}
}
In this example, the Surface wraps a Text composable and applies a rounded corner shape, elevation, and color.
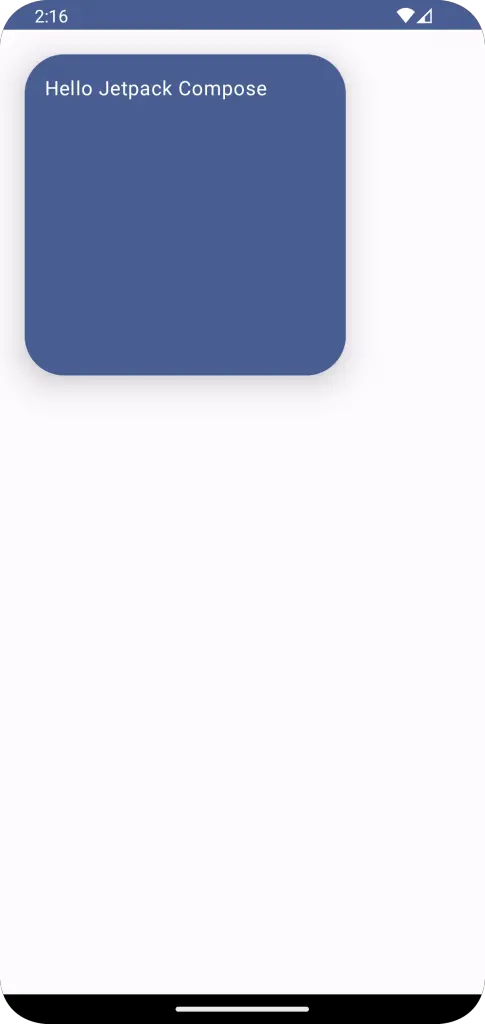
Differences Between Box and Surface
- Layering and Alignment: Box is primarily used to stack its children, allowing layering of composables on top of each other. On the other hand, Surface doesn’t stack its children. Instead, it acts as a single-child layout composable.
- Material Design Styles: Surface automatically applies Material Design styles such as elevation, shape, and color. It also adapts to light/dark theme modes. Box, in contrast, does not provide these features out of the box.
- Usage: While Box is mainly used for alignment and layering, Surface is typically used as a container for other composables, where you wish to apply Material Design aesthetics.
It’s also worth mentioning that Box and Surface can be used together. For example, a Surface could be used as a container to apply material design styles, and within that Surface, a Box could be used to layer or align its children.
By understanding the differences between Box and Surface, you can leverage the unique features of each to design more diverse and aesthetically pleasing UIs in your Jetpack Compose applications.