How to Add Border to Surface in Android Jetpack Compose
Android’s Jetpack Compose allows us to create UI elements with ease, and Surface is a versatile composable that we can use for multiple purposes, such as drawing backgrounds, handling themes, applying elevations, and more.
In this blog post, we will learn how to add a border to a Surface in Jetpack Compose. We can create Border using BorderStroke and border parameter of Surface composable.
@Composable
fun SurfaceExample() {
Surface(
modifier = Modifier.padding(16.dp).fillMaxSize(),
color = Color.White,
border = BorderStroke(2.dp, color = Color.Red),
) {
Text(
text = "Hello, Jetpack Compose!",
modifier = Modifier.padding(16.dp)
)
}
}
In the example above, the Surface composable contains a Text composable. We’ve assigned the border to the Surface using the border parameter.
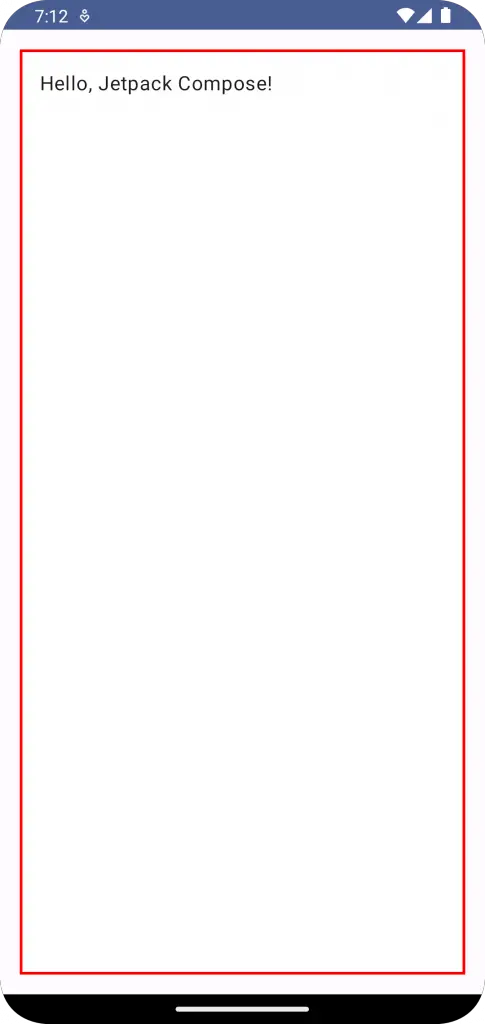
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.BorderStroke
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
SurfaceExample()
}
}
}
}
}
@Composable
fun SurfaceExample() {
Surface(
modifier = Modifier.padding(16.dp).fillMaxSize(),
color = Color.White,
border = BorderStroke(2.dp, color = Color.Red),
) {
Text(
text = "Hello, Jetpack Compose!",
modifier = Modifier.padding(16.dp)
)
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
SurfaceExample()
}
}
And that’s it! You’ve successfully added a border to a Surface in Jetpack Compose. Feel free to play around with different border widths and colors to suit your design needs.