How to Set Icon Background Color in Android Jetpack Compose
Icons are an integral part of mobile app development, assisting users in navigating your app with ease. While using Jetpack Compose, you may want to customize your icons further to fit your app’s design theme.
In this blog post, we will show you how to set an icon’s background color in Jetpack Compose.
How to Use Icon Composable
The Icon composable in Jetpack Compose is a convenient tool for drawing icons on your screen. It follows Material Design guidelines and offers customization options for size, tint, and more.
Icon(
imageVector = Icons.Default.Home,
contentDescription = "Home Icon"
)
This example uses the Icon composable to display the default home icon.
How to Set Icon Background Color
To set an icon’s background color, you can use the Modifier.background function. It takes in a color and applies it as a background to the composable.
Here’s how you can add a background color to your icon.
@Composable
fun IconExample() {
Column {
Icon(
imageVector = Icons.Default.Home,
contentDescription = "Home Icon",
modifier = Modifier.background(color = Color.Yellow)
.size(72.dp)
)
}
}
In this example, the Modifier.background function applies a yellow background to the home icon. This change makes the icon stand out more and can help to attract the user’s attention.
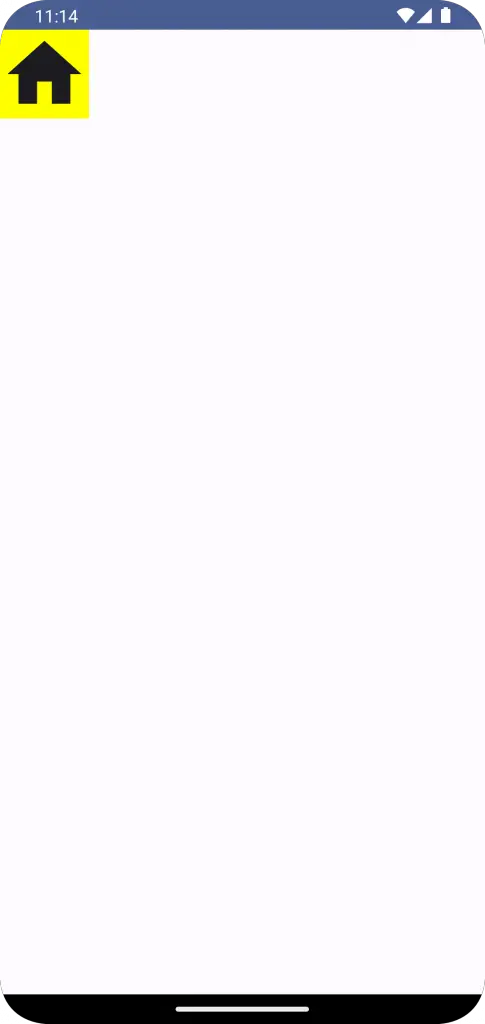
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.size
import androidx.compose.material.icons.Icons
import androidx.compose.material.icons.filled.Home
import androidx.compose.material3.Icon
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
IconExample()
}
}
}
}
}
@Composable
fun IconExample() {
Column{
Icon(
imageVector = Icons.Default.Home,
contentDescription = "Home Icon",
modifier = Modifier.background(color = Color.Yellow)
.size(72.dp)
)
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
IconExample()
}
}
Customizing your icons with background colors is an effective way to enhance the aesthetic of your app and provide a more engaging user experience. Jetpack Compose makes this task straightforward and flexible.