How to Create Horizontal List with LazyRow in Android Jetpack Compose
In Android development, creating a horizontal list was traditionally done using RecyclerView with a LinearLayoutManager set to horizontal orientation. With Jetpack Compose’s introduction, this process is made simpler and more intuitive with LazyRow.
In this blog post, we will learn how to create a horizontal list using the LazyRow Composable.
To create a horizontal list with LazyRow, we need some data. For simplicity, we’ll use a list of integers. We’ll also apply some basic styling to each item:
import androidx.compose.foundation.lazy.LazyRow
import androidx.compose.foundation.lazy.items
@Composable
fun HorizontalListView() {
val items = (1..50).toList()
LazyRow(contentPadding = PaddingValues(horizontal = 10.dp, vertical = 20.dp)) {
items(items) { item ->
Text(
text = "Item $item",
modifier = Modifier
.padding(end = 10.dp)
.background(color = Color.LightGray)
.padding(16.dp)
)
}
}
}
Here, LazyRow creates a horizontally scrolling list. The items lambda function is responsible for generating the UI for each item in the list. In our case, we are just showing a Text Composable for each item.
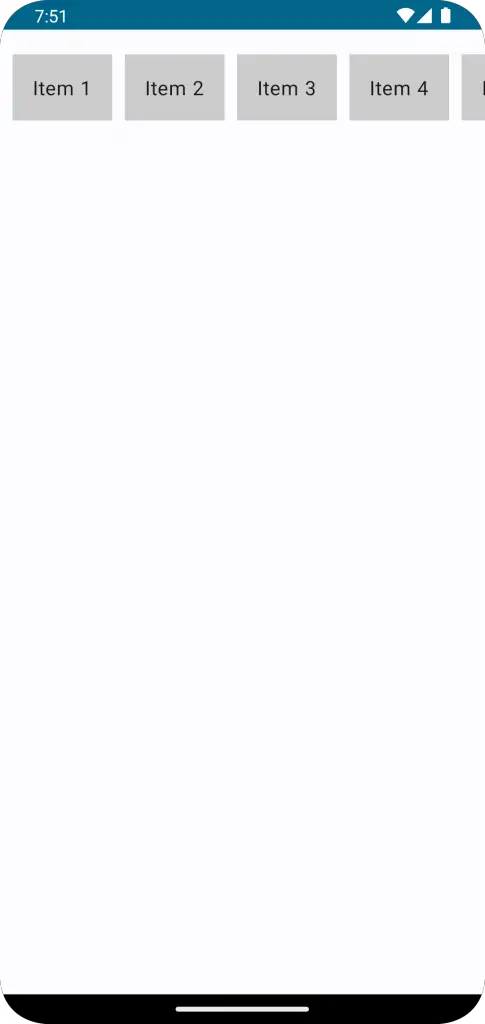
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.lazy.items
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
import androidx.compose.foundation.layout.PaddingValues
import androidx.compose.foundation.lazy.LazyRow
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
HorizontalListView()
}
}
}
}
}
@Composable
fun HorizontalListView() {
val items = (1..50).toList()
LazyRow(contentPadding = PaddingValues(horizontal = 10.dp, vertical = 20.dp)) {
items(items) { item ->
Text(
text = "Item $item",
modifier = Modifier
.padding(end = 10.dp)
.background(color = Color.LightGray)
.padding(16.dp)
)
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
HorizontalListView()
}
}
You now know how to create a horizontal list using LazyRow in Jetpack Compose. With this knowledge, you can create various types of horizontal lists and carousels.
One Comment