How to Scale Shapes in Jetpack Compose
Jetpack Compose provides various tools to easily scale and transform composable UI elements. In this blog post, we’ll look at how to scale shapes drawn on a Canvas using Compose’s scale modifier.
Basic Scaling
Here is a composable that draws a rectangle on a Canvas and scales it non-uniformly using scale
:
@Composable
fun ScaledRectangle() {
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
scale(scaleX = 2f, scaleY = 1.5f) {
drawRect(
color = Color.Red,
topLeft = Offset(x = canvasWidth / 4, y = canvasHeight / 4),
size = Size(width = 500f, height = 500f)
)
}
}
}
Breaking this down:
- Get the Canvas dimensions using
size.width
andsize.height
- Apply non-uniform scaling via
scale()
- Scale x-axis by 2x
- Scale y-axis by 1.5x
- Draw the rectangle
- Position is based on original Canvas size
- Size is specified before scaling is applied
This scales the rectangle to be wider than it is tall.
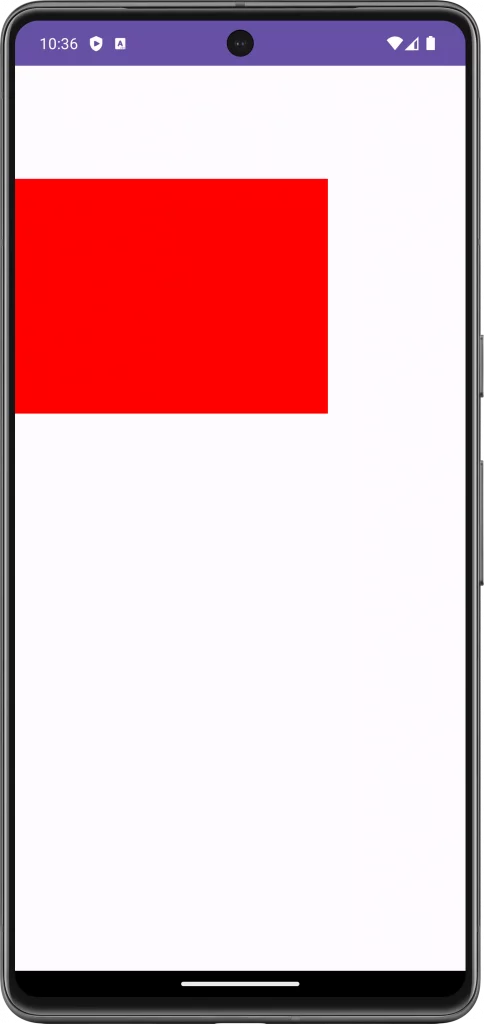
Custom Scaling
We can also manually adjust the rectangle’s position and size based on scale factors:
@Composable
fun ManuallyScaledRectangle() {
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
val scaleX = 2f
val scaleY = 2f
drawRect(
color = Color.Red,
topLeft = Offset(
x = canvasWidth / 4 * scaleX,
y = canvasHeight / 4 * scaleY
),
size = Size(
width = 200f * scaleX,
height = 200f * scaleY
)
)
}
}
Here we:
- Calculate scale factors
- Adjust top-left position by scale factors
- Adjust size by scale factors
This gives complete control over scaling behavior.
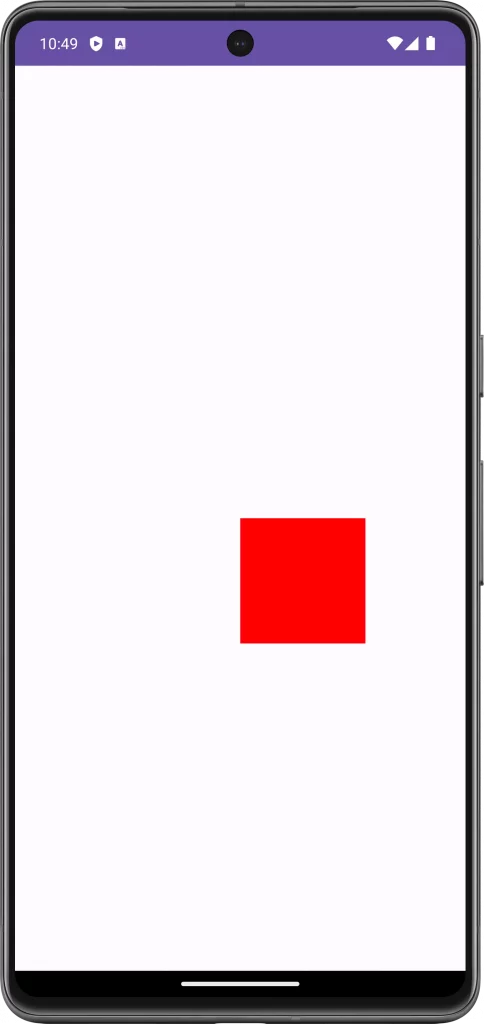
Scaling shapes in Jetpack Compose is a straightforward yet effective way to create dynamic and responsive UIs. By understanding the Canvas API and how to apply transformations like scaling, you can enhance the visual appeal of your Android app and create a more engaging user experience.
2 Comments