How to Draw an Image on Canvas in Jetpack Compose
In Android app development, visual elements like images play a pivotal role in enhancing user interfaces. Jetpack Compose, with its versatile UI toolkit, allows for an array of creative possibilities, including drawing images on a canvas.
This capability is particularly useful for custom UIs that require more than just standard image views. In this blog post, we’ll explore how to draw an image on a canvas in Jetpack Compose, using a simple example.
Canvas in Jetpack Compose provides a playground for custom drawings, including shapes, text, and images. It’s a powerful tool for developers looking to create unique graphics or incorporate images in custom layouts.
Example: Draw an Image on Canvas
Let’s examine how to draw an image on a canvas in Jetpack Compose:
First, we set up a Canvas
composable that fills the maximum available size. This serves as our drawing area.
@Composable
fun Example() {
Canvas(modifier = Modifier.fillMaxSize(), onDraw = {
// Image drawing code will be placed here
})
}
Load and Draw the Image
In this example, we load an image and draw it on the canvas. We use ImageBitmap.imageResource
to load an image resource.
val catImage = ImageBitmap.imageResource(id = R.drawable.cat)
Canvas(modifier = Modifier.fillMaxSize(), onDraw = {
drawImage(catImage)
})
In this code:
val catImage = ImageBitmap.imageResource(id = R.drawable.cat)
: This line loads an image resource (in this case, a cat image) into anImageBitmap
. TheR.drawable.cat
refers to the resource identifier of the cat image in your drawable resources.drawImage(catImage)
: Inside theonDraw
lambda of theCanvas
, this function draws the loaded image onto the canvas.
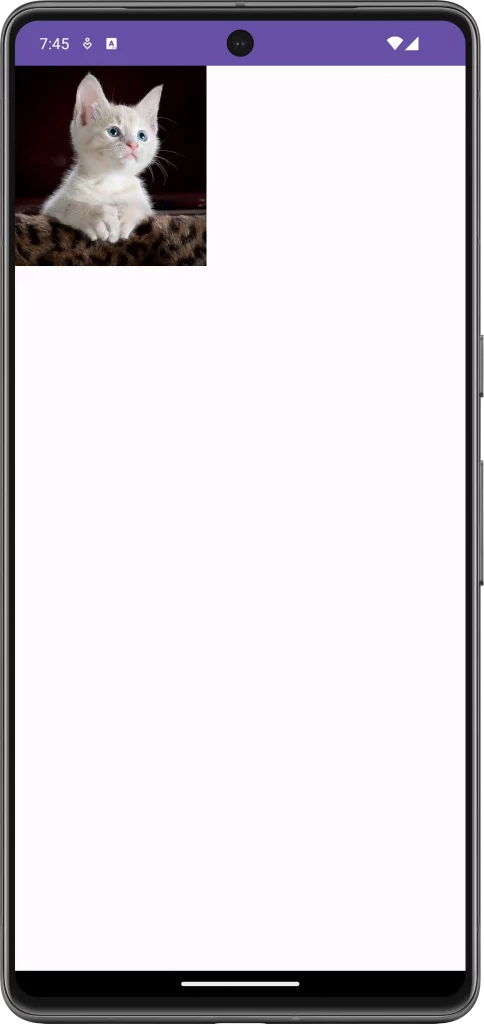
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Canvas
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.ImageBitmap
import androidx.compose.ui.res.imageResource
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
Example()
}
}
}
}
}
}
@Composable
fun Example() {
val catImage = ImageBitmap.imageResource(id = R.drawable.cat)
Canvas(modifier = Modifier.fillMaxSize(), onDraw = {
drawImage(catImage)
})
}
Customize the Image Rendering
Resize and Position the Image
If you need to resize or position the image on the canvas, you can use additional parameters in the drawImage
function.
drawImage(
image = catImage,
dstSize = IntSize(width = 300, height = 300), // Resizes the image
dstOffset = IntOffset(x = 100, y = 200) // Positions the image
)
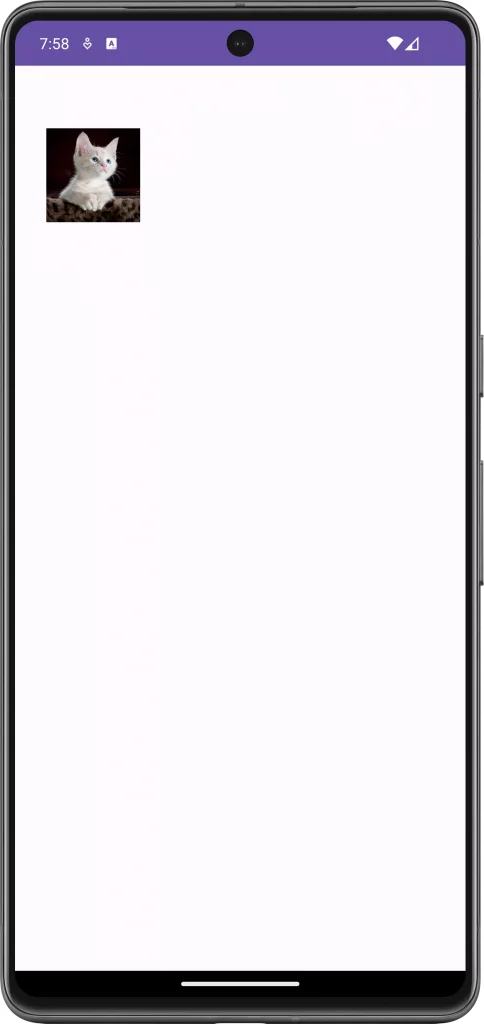
Apply Transformations
You can apply transformations like scaling, rotation, or translation to the canvas before drawing the image, allowing for more complex layouts and effects.
Drawing an image on a canvas in Jetpack Compose opens up numerous possibilities for creative UI design in Android apps. By understanding how to load and render images on the canvas, you can craft visually striking interfaces that stand out.