How to change Background Color of Row and Column in Android Jetpack Compose
Row and Column are two important composables to create a mobile app layout using Jetpack Compose. Row composable helps to arrange the content horizontally whereas Column arranges child elements vertically. Let’s check how to change the default background color of Row and Column in Jetpack Compose.
Jetpack Compose Row Background Color
You can customize the Row background color using Modifier.background(). See the code snippet given below where the yellow color is given as the background color of the Row.
@Composable
fun RowExample() {
Row(modifier = Modifier
.fillMaxWidth()
.height(200.dp)
.padding(20.dp)
.background(Color.Yellow))
{
Text(text = "Row background color example", fontSize = 24.sp)
}
}
Following is the output.
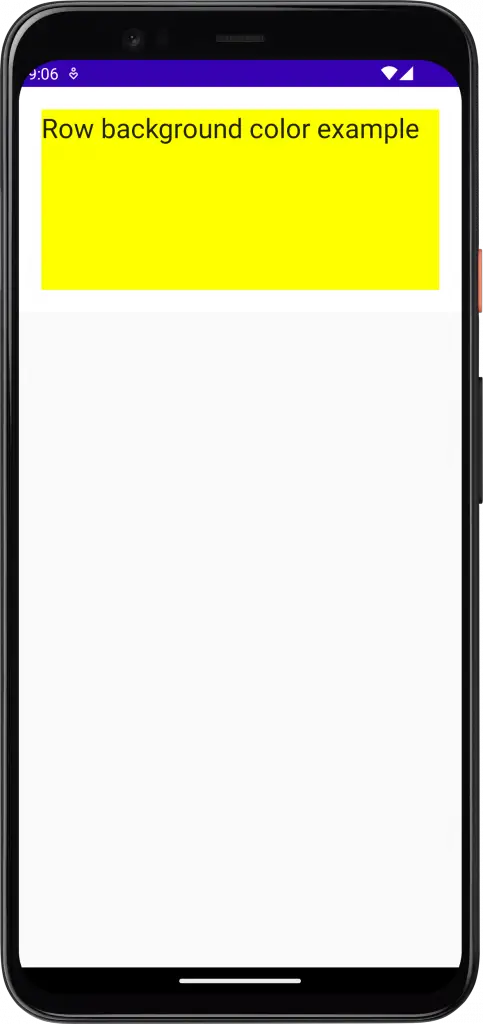
Following is the complete code for reference.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.Row
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
RowExample()
}
}
}
}
}
}
@Composable
fun RowExample() {
Row(modifier = Modifier
.fillMaxWidth()
.height(200.dp)
.padding(20.dp)
.background(Color.Yellow))
{
Text(text = "Row background color example", fontSize = 24.sp)
}
}
Jetpack Compose Column Background Color
Similarly, you can change the background color of the Column using Modifier.background() function. The code snippet is given below.
@Composable
fun ColumnExample() {
Column(modifier = Modifier
.fillMaxWidth()
.height(300.dp)
.padding(20.dp)
.background(Color.Cyan))
{
Text(text = "Column background color example", fontSize = 24.sp)
}
}
And you will get the following output.
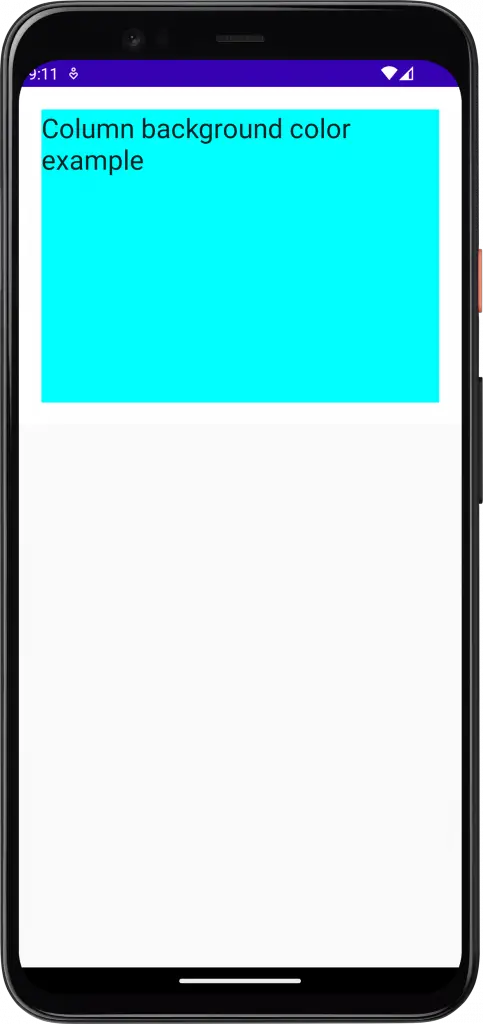
Following is the complete code.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
ColumnExample()
}
}
}
}
}
}
@Composable
fun ColumnExample() {
Column(modifier = Modifier
.fillMaxWidth()
.height(300.dp)
.padding(20.dp)
.background(Color.Cyan))
{
Text(text = "Column background color example", fontSize = 24.sp)
}
}
That’s how you change the background color of Rows and Columns in Jetpack Compose.