How to Translate Position of Shapes in Jetpack Compose
Creating dynamic and interactive user interfaces in Android applications involves not just drawing shapes but also manipulating their positions. Jetpack Compose, with its modern UI toolkit, provides a flexible canvas for such custom drawings. In this context, translating the position of shapes is a fundamental aspect.
This blog post will discuss how to translate or move shapes in Jetpack Compose, using the example of moving a circle.
Translation in Jetpack Compose
Translation in graphical terms refers to moving an object from one place to another. In Jetpack Compose, this can be achieved within the Canvas
composable using the translate
function. This function shifts the origin of the canvas, thereby changing the position where shapes are drawn.
Within the Canvas
, we can use the translate
function to move our drawing code to a different position. Here’s an example of translating the position of a circle:
@Composable
fun Example() {
Canvas(modifier = Modifier.fillMaxSize()) {
translate(left = 200f, top = -300f) {
drawCircle(Color.Blue, radius = 50.dp.toPx())
}
}
}
In this example:
translate(left = 200f, top = -300f)
: This line moves the drawing context 200 pixels to the right and 300 pixels upwards. The parametersleft
andtop
denote the horizontal and vertical displacement, respectively.drawCircle(...)
: Draws a blue circle at the translated position. The radius is set to 50 density-independent pixels, converted to pixels usingtoPx()
.
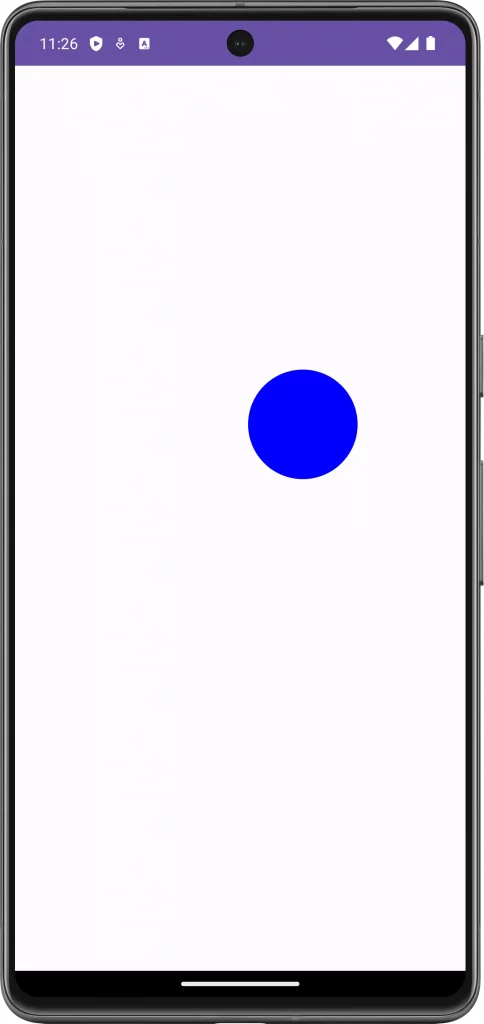
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Canvas
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.graphics.drawscope.translate
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Example()
}
}
}
}
}
@Composable
fun Example() {
Canvas(modifier = Modifier.fillMaxSize()) {
translate(left = 200f, top = -300f) {
drawCircle(Color.Blue, radius = 50.dp.toPx())
}
}
}
Customize the Translation
Adjust Translation Values
You can modify the left
and top
values in the translate
function to control the exact position of the shape. Positive values move the shape right and down, while negative values move it left and up.
Combine with Other Transformations
Translation can be combined with other transformations like scaling or rotation to create more complex graphical effects.
Translating the position of shapes in Jetpack Compose is a simple yet powerful tool in the UI developer’s arsenal. It allows for dynamic and interactive UI designs, giving developers the freedom to create custom and engaging user experiences.
By mastering translation and other canvas transformations, you can bring a new level of creativity to your Android apps.
One Comment