How to Add Hyperlink in Android Jetpack Compose
Hyperlinks provide a convenient way to guide users to different parts of your app or external websites. This is incredibly useful in cases such as guiding users to your terms and conditions, privacy policy, or perhaps citing a source.
In this tutorial, we will discuss how you can create hyperlinks within a sentence that can be clicked to open a URL using Jetpack Compose, Google’s modern toolkit for building native Android UIs.
The traditional Android View system allows you to create hyperlinks using TextView and SpannableString. However, Jetpack Compose simplifies this process by providing AnnotatedString and ClickableText composables.
AnnotatedString is a powerful tool that allows you to create complex, styled, and interactive text, while ClickableText makes this interactive text clickable and allows you to specify actions on click events.
Here’s an example of creating a sentence with a clickable hyperlink using these two composables:
@Composable
fun HyperlinkInSentenceExample() {
val sourceText = "Check out my website: "
val hyperlinkText = "CodingWithRashid"
val endText = " for more awesome content."
val uri = "https://www.codingwithrashid.com"
val annotatedString = buildAnnotatedString {
append(sourceText)
withStyle(style = SpanStyle(textDecoration = TextDecoration.Underline, color = Color.Blue)) {
append(hyperlinkText)
addStringAnnotation(
tag = "URL",
annotation = uri,
start = length - hyperlinkText.length,
end = length
)
}
append(endText)
}
val uriHandler = LocalUriHandler.current
ClickableText(
text = annotatedString,
onClick = { offset ->
annotatedString.getStringAnnotations(tag = "URL", start = offset, end = offset)
.firstOrNull()?.let { annotation ->
uriHandler.openUri(annotation.item)
}
}
)
}
In this example, we build a sentence in parts using an AnnotatedString builder. The hyperlinkText is annotated with a URL and styled with SpanStyle to give it an underline and a different color. The ClickableText composable then makes this entire sentence clickable. When the user clicks on the hyperlink, it retrieves the annotation and opens the URL.
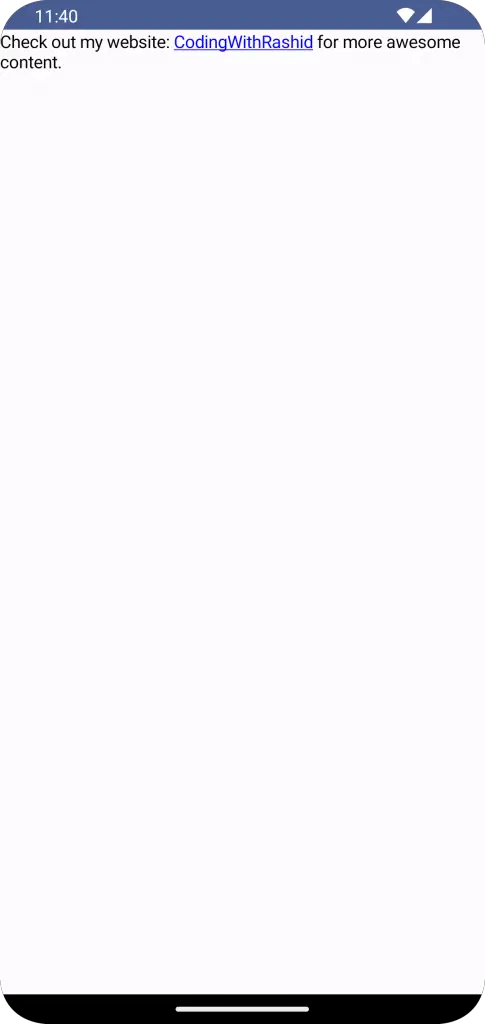
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.text.ClickableText
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.platform.LocalUriHandler
import androidx.compose.ui.text.SpanStyle
import androidx.compose.ui.text.buildAnnotatedString
import androidx.compose.ui.text.style.TextDecoration
import androidx.compose.ui.text.withStyle
import androidx.compose.ui.tooling.preview.Preview
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
HyperlinkInSentenceExample()
}
}
}
}
}
@Composable
fun HyperlinkInSentenceExample() {
val sourceText = "Check out my website: "
val hyperlinkText = "CodingWithRashid"
val endText = " for more awesome content."
val uri = "https://www.codingwithrashid.com"
val annotatedString = buildAnnotatedString {
append(sourceText)
withStyle(style = SpanStyle(textDecoration = TextDecoration.Underline, color = Color.Blue)) {
append(hyperlinkText)
addStringAnnotation(
tag = "URL",
annotation = uri,
start = length - hyperlinkText.length,
end = length
)
}
append(endText)
}
val uriHandler = LocalUriHandler.current
ClickableText(
text = annotatedString,
onClick = { offset ->
annotatedString.getStringAnnotations(tag = "URL", start = offset, end = offset)
.firstOrNull()?.let { annotation ->
uriHandler.openUri(annotation.item)
}
}
)
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
HyperlinkInSentenceExample()
}
}
Creating interactive text with hyperlinks within sentences has never been easier with Jetpack Compose’s AnnotatedString and ClickableText composables.
This approach provides a simpler and more flexible way to handle hyperlinks, whether you’re displaying terms of service, citing sources, or guiding users to different parts of your app.