How to Get Canvas Size in Jetpack Compose
In the versatile world of Android app development, Jetpack Compose has redefined how developers approach UI creation. A significant aspect of this toolkit is the Canvas composable, a powerful tool for custom drawings and graphics.
Understanding the size of the canvas is critical for creating responsive and dynamic designs. This blog post will focus on how to obtain the canvas size in Jetpack Compose and its implications in custom UI development.
Canvas in Jetpack Compose
Canvas in Jetpack Compose provides a drawing area where developers can create custom graphics, shapes, and animations. Knowing the canvas size is essential for scaling these elements appropriately, ensuring they fit well within the UI, regardless of device size or screen orientation.
Obtain Canvas Size in Jetpack Compose
In Jetpack Compose, the size of the canvas can be determined within the drawing context. Here’s how you can obtain the width and height of the canvas:
@Composable
fun ExampleCanvas() {
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
// Custom drawing code using canvasWidth and canvasHeight
}
}
In this example:
Canvas(modifier = Modifier.fillMaxSize())
: Sets up a full-size canvas.val canvasWidth = size.width
: Retrieves the width of the canvas.val canvasHeight = size.height
: Retrieves the height of the canvas.
The size
property within the Canvas
scope provides the dimensions of the canvas, which can then be used for drawing and layout calculations.
Utilize Canvas Size in Custom Drawings
With the canvas size known, you can create responsive designs that adapt to different screen sizes and orientations.
Use the canvas dimensions to position elements dynamically. For example, to place a circle at the center of the canvas:
@Composable
fun ExampleCanvas() {
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
val centerX = canvasWidth / 2
val centerY = canvasHeight / 2
drawCircle(Color.Black, center = Offset(centerX, centerY), radius = 250f)
}
}
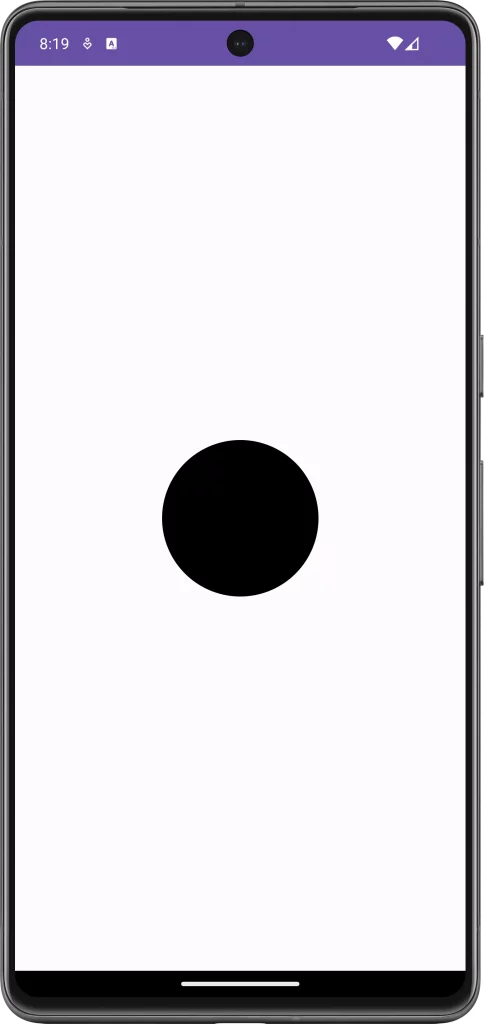
Understanding and utilizing the canvas size in Jetpack Compose is vital for creating responsive, dynamic, and visually appealing custom UI components. By properly leveraging the canvas dimensions, developers can craft sophisticated designs that seamlessly adapt to varying screen sizes and orientations.
One Comment