How to Set Gradient Background for TopAppBar in Android Jetpack Compose
Android’s Jetpack Compose is transforming the landscape of UI development with its declarative approach. Among its composables, the TopAppBar stands as an essential tool for creating action bars or app bars.
Although changing the background color of the TopAppBar to a solid color is quite simple, making it a gradient color adds a certain visual appeal to your app. This tutorial will guide you on setting a gradient background for the TopAppBar using Jetpack Compose.
How to Define Gradient in Jetpack Compose
The first step is to define the gradient colors. In Jetpack Compose, you can use the Brush.horizontalGradient() function to create a horizontal gradient. Here is how to define a gradient transitioning from Cyan to Green:
Brush.horizontalGradient(
colors = listOf(Color.Cyan, Color.Green)
)
This code defines a linear gradient that transitions horizontally from Cyan to Green.
How to Create Gradient TopAppBar in Jetpack Compose
Now that we have our gradient, let’s create a TopAppBar with this gradient as its background. Here is the code:
@Composable
fun AppBarExample() {
Scaffold(
topBar = {
TopAppBar(
modifier = Modifier.background(
Brush.horizontalGradient(
colors = listOf(
Color.Cyan,
Color.Green
)
)
),
title = {
Text("My App")
},
colors = TopAppBarDefaults.smallTopAppBarColors(containerColor = Color.Transparent )
)
}
) { contentPadding ->
// Screen content
Text(text = "Jetpack Compose App Bar Example",
modifier = Modifier.padding(contentPadding))
}
}
Here’s what’s going on:
We use the TopAppBar composable and assign the gradient we created to its background using the Modifier.background function.
The TopAppBarDefaults.smallTopAppBarColors is used to specify the color scheme for our app bar. We set the containerColor to Color.Transparent to make the gradient visible.
Following is the output:
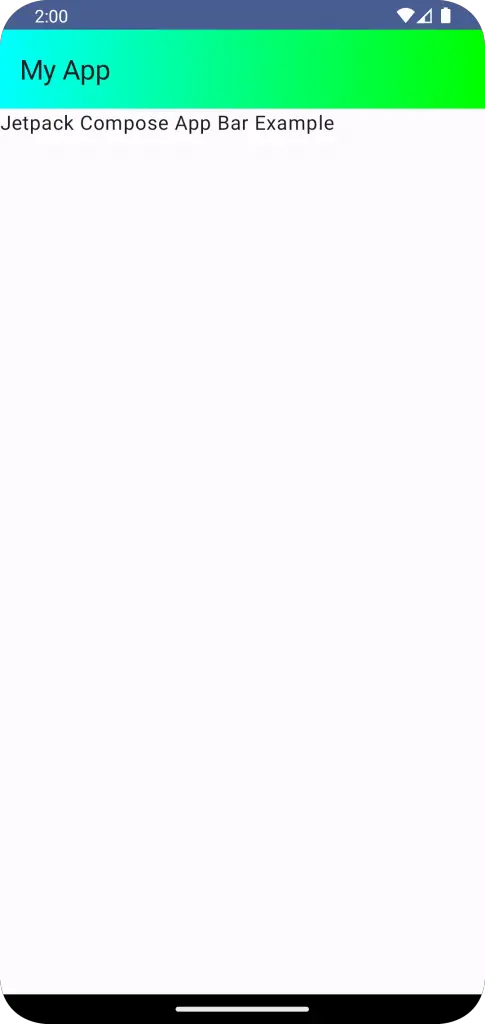
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TopAppBar
import androidx.compose.material3.TopAppBarDefaults
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Brush
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
AppBarExample()
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun AppBarExample() {
Scaffold(
topBar = {
TopAppBar(
modifier = Modifier.background(
Brush.horizontalGradient(
colors = listOf(
Color.Cyan,
Color.Green
)
)
),
title = {
Text("My App")
},
colors = TopAppBarDefaults.smallTopAppBarColors(containerColor = Color.Transparent )
)
}
) { contentPadding ->
// Screen content
Text(text = "Jetpack Compose App Bar Example",
modifier = Modifier.padding(contentPadding))
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
AppBarExample()
}
}
Even though Jetpack Compose doesn’t provide a built-in feature to directly set a gradient background for the TopAppBar, we can use the power of modifiers and brushes to create beautiful gradient effects. This can significantly enhance the visual appeal of your app and provide a more engaging user experience.
One Comment