How to Set Image Background for TopAppBar in Android Jetpack Compose
The TopAppBar is a composable that provides a container for toolbar content at the top of the screen. While setting a solid color or a gradient as the background of TopAppBar is common, you might find yourself needing to set an image as the background.
This can help create a more engaging and visually appealing interface. In this post, we’ll show you how to set an image background for TopAppBar in Jetpack Compose.
Step 1: Import Your Image
Before we start, you’ll need to have the image you want to use in your res/drawable
directory. If you haven’t done this already, copy your image file into this directory. For the purpose of this tutorial, let’s assume the image is named my_image
.
See this tutorial to learn how to import images using Android Studio.
Step 2: Create the Image Background TopAppBar
Just like when setting a gradient background, we can’t directly set an image as the TopAppBar’s background. Instead, we’ll use a Box to layer the TopAppBar over an Image composable. Here’s how you can do this:
@Composable
fun AppBarExample() {
Scaffold(
topBar = {
Box(
modifier = Modifier.fillMaxWidth()
) {
Image(
painter = painterResource(id = R.drawable.my_image),
contentDescription = "App Bar Background",
contentScale = ContentScale.Crop,
modifier = Modifier
.fillMaxWidth()
.height(56.dp)
)
TopAppBar(
title = {
Text("My App")
},
colors = TopAppBarDefaults.smallTopAppBarColors(containerColor = Color.Transparent)
)
}
}
) { contentPadding ->
// Screen content
Text(text = "Jetpack Compose App Bar Example",
modifier = Modifier.padding(contentPadding))
}
}
A Box composable is used to allow the layering of composables. Inside the Box, an Image composable is used to display the image, which is loaded using painterResource(id = R.drawable.my_image). The contentScale parameter is set to ContentScale.Crop to make the image scale correctly inside the app bar.
The TopAppBar is then layered on top of the Image. Its background color is set to Color.Transparent so that the image can be seen.
Following is the output.
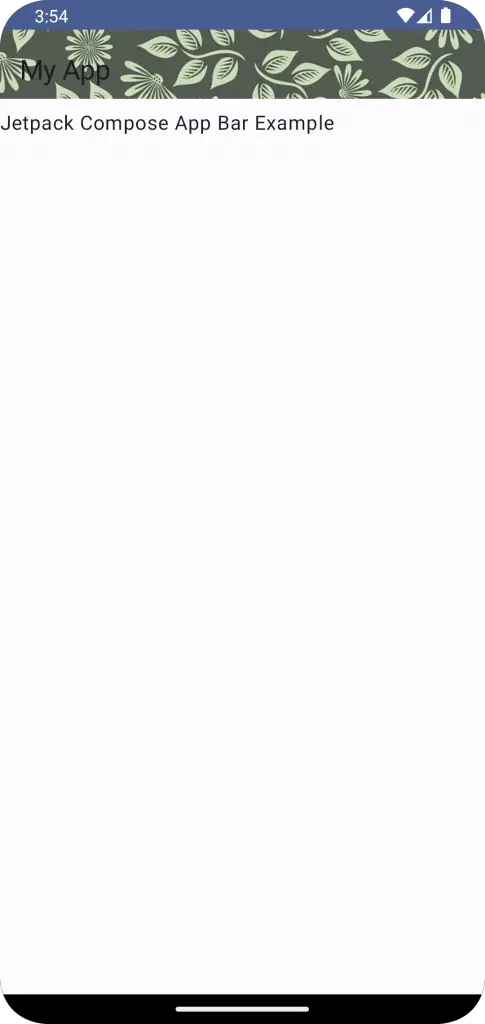
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TopAppBar
import androidx.compose.material3.TopAppBarDefaults
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.layout.ContentScale
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
AppBarExample()
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun AppBarExample() {
Scaffold(
topBar = {
Box(
modifier = Modifier.fillMaxWidth()
) {
Image(
painter = painterResource(id = R.drawable.my_image),
contentDescription = "App Bar Background",
contentScale = ContentScale.Crop,
modifier = Modifier
.fillMaxWidth()
.height(56.dp)
)
TopAppBar(
title = {
Text("My App")
},
colors = TopAppBarDefaults.smallTopAppBarColors(containerColor = Color.Transparent)
)
}
}
) { contentPadding ->
// Screen content
Text(text = "Jetpack Compose App Bar Example",
modifier = Modifier.padding(contentPadding))
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
AppBarExample()
}
}
While Jetpack Compose doesn’t offer a direct way to set an image as the TopAppBar background, this limitation can be overcome with the use of the Box composable. By layering an Image composable beneath the TopAppBar, we can create visually engaging app bars with image backgrounds.