How to Show Image from URL in Android Jetpack Compose
Jetpack Compose has transformed Android UI development, making it quicker and more efficient. In this enhanced guide, you’ll learn not just how to display an image from a URL using Jetpack Compose, but also how to optimize it for better performance.
We’ll utilize the Coil library to achieve this, which is a powerful tool for image loading in Android.
Why Use Jetpack Compose?
Jetpack Compose offers a modern way to build UIs for Android apps. It’s easier to work with and saves a lot of time. Plus, it’s highly customizable. So, it’s a great choice for building top-notch UIs quickly.
Why Coil?
Coil stands for “Co-routine Image Loader,” and it’s designed specifically for Android. This Kotlin-first image-loading library allows for hassle-free image loading while offering advanced features like image caching.
Add Dependencies
First, you’ll need to add the required Coil dependency to your build.gradle
(Module: App) file. Make sure the dependency is placed within the dependencies {}
block. Always double-check for the latest version of the library.
implementation("io.coil-kt:coil-compose:2.4.0")
Set Up Permissions
Before you go ahead, don’t forget to add Internet permission to your Android Manifest file. Here’s how:
<uses-permission android:name="android.permission.INTERNET" />
Writing the Code
Coil makes it possible to load images asynchronously in Jetpack Compose. The AsyncImage
composable from Coil serves this purpose well. Below is a simple example code snippet to demonstrate this.
import coil.compose.AsyncImage
AsyncImage(
model = "https://cdn.pixabay.com/photo/2021/11/12/12/16/leaves-6788800_1280.jpg",
contentDescription = null
)
Display Images with Fixed Dimensions
In some cases, you might want to display an image with fixed dimensions. This is especially useful when you want to control how much space the image takes up on the screen. In Jetpack Compose with Coil, you can achieve this quite easily. Below, we’ll go over how to set a fixed width and height for the image.
Code for Fixed Dimensions
Adding fixed dimensions to your AsyncImage
is simple. You just need to use the Modifier.size()
function. Here’s a sample code snippet:
import androidx.compose.ui.unit.dp
import androidx.compose.ui.Modifier
import coil.compose.AsyncImage
AsyncImage(
model = "https://cdn.pixabay.com/photo/2021/11/12/12/16/leaves-6788800_1280.jpg",
contentDescription = null,
modifier = Modifier.size(200.dp, 200.dp)
)
In this example, both the width and height are set to 200 density-independent pixels (dp). You can adjust these dimensions as per your requirement.
Complete Code
Here is a complete code example that incorporates everything you’ve learned so far.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.size
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import coil.compose.AsyncImage
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
imageExample()
}
}
}
}
}
@Composable
fun imageExample() {
Box() {
AsyncImage(
model = "https://cdn.pixabay.com/photo/2021/11/12/12/16/leaves-6788800_1280.jpg",
contentDescription = null,
modifier = Modifier.size(200.dp, 200.dp)
)
}
}
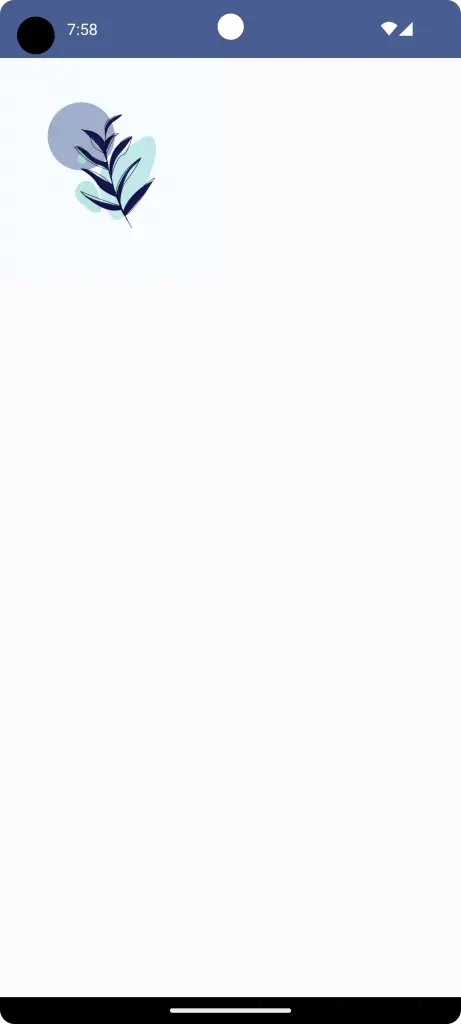
That’s how you can load and display an image from a URL in Jetpack Compose using the Coil library. This approach is efficient, easy to implement, and optimized for Android development.
One Comment