How to Add TextField in Android Jetpack Compose
Adding a TextField to your Android app is a critical step for getting user input. This blog post aims to guide you through integrating a TextField in Android Jetpack Compose seamlessly.
What is a TextField?
A TextField is a basic UI element that allows users to enter text. It’s essential for gathering data like usernames, passwords, and more.
Prerequisites
Before diving in, make sure you have Android Studio installed and are familiar with Kotlin.
Why Use Jetpack Compose?
Jetpack Compose is Android’s modern UI toolkit to help you build native interfaces. It simplifies the UI development process and makes it more enjoyable.
Add a Basic TextField in Jetpack Compose
The first thing you need to know is how to add a simple TextField. Below is the code snippet for that.
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput, onValueChange = {textInput = it},
modifier = Modifier.fillMaxWidth().padding(10.dp))
}
The value of the TextField is stored in the textInput
variable. To resolve any errors in Android Studio, use the option + return (or alt + enter) shortcut to import the necessary functions like getValue
and setValue
.
To have a working TextField, you need to manage its state. Jetpack Compose makes this easy with its built-in state management.
Full Example Code
Here is the example code.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.TextField
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextFieldExample()
}
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput, onValueChange = {textInput = it},
modifier = Modifier
.fillMaxWidth()
.padding(10.dp))
}
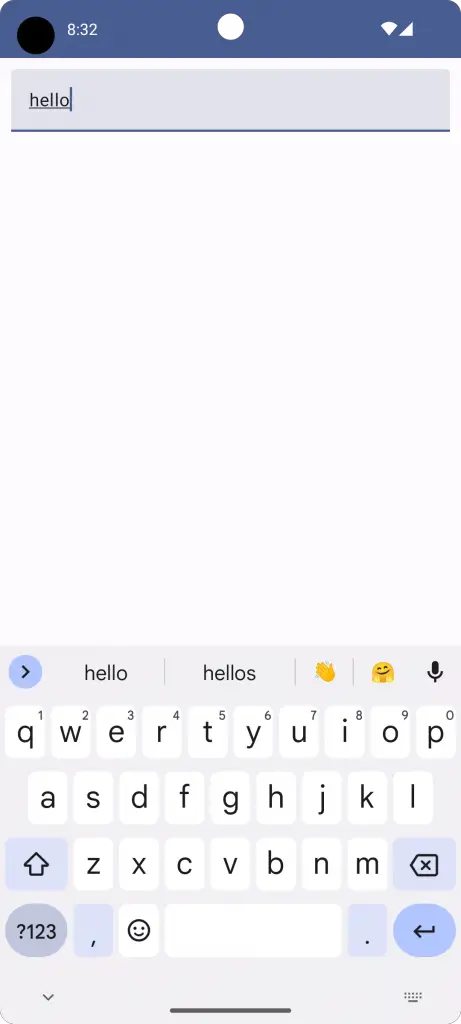
Customize Your TextField
TextFields aren’t one-size-fits-all. Let’s explore how to customize them further.
Placeholder Text
Use the placeholder parameter to display TextField hint text in Jetpack Compose.
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput, onValueChange = {textInput = it},
placeholder = { Text("Enter text") },
modifier = Modifier
.fillMaxWidth()
.padding(10.dp))
}
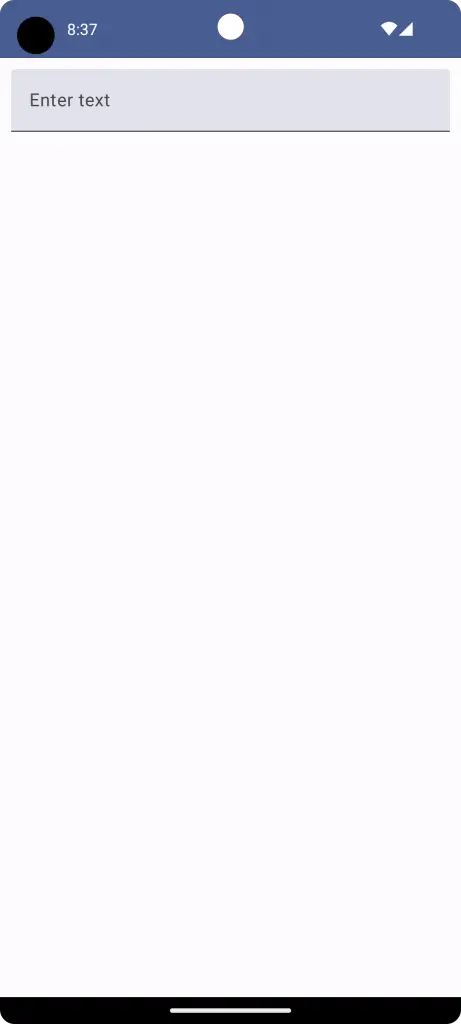
Keyboard Options
Control the keyboard type using keyboardOptions. For example, let’s make the TextField number only in the following snippet.
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput, onValueChange = {textInput = it},
placeholder = { Text("Enter text") },
keyboardOptions = KeyboardOptions(keyboardType = KeyboardType.Number),
modifier = Modifier
.fillMaxWidth()
.padding(10.dp))
}
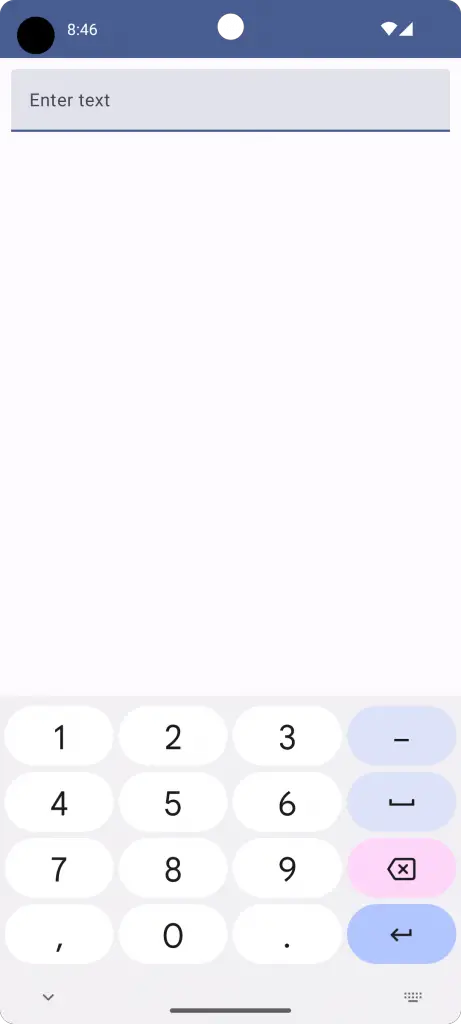
Styling TextFields
You can also style your TextField with custom colors, shapes, and more. For example, you can change TextField background color as given below.
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput, onValueChange = {textInput = it},
colors = TextFieldDefaults.textFieldColors(containerColor = Color.Yellow),
modifier = Modifier
.fillMaxWidth()
.padding(10.dp))
}
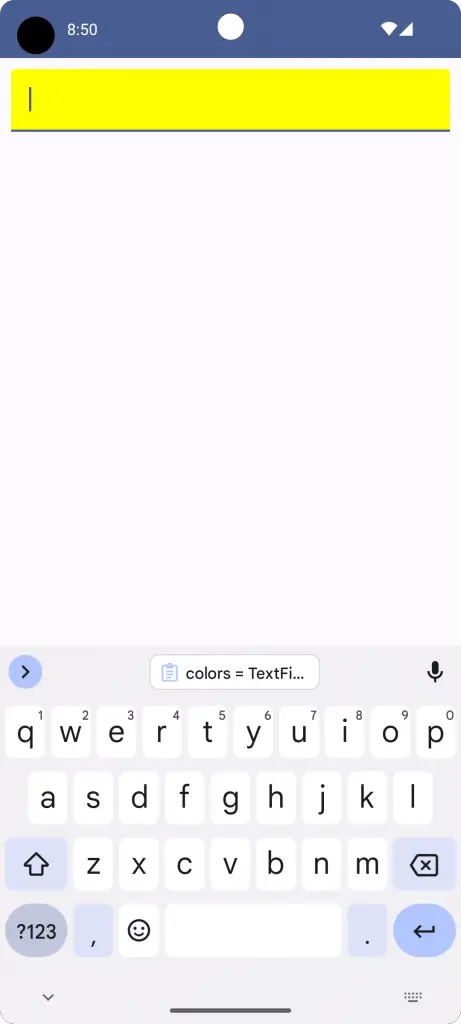
There you have it! You now know how to add a TextField in Android Jetpack Compose and manage its state. State management is crucial, and Jetpack Compose makes it hassle-free.
8 Comments