How to Create a Simple Card in Android Jetpack Compose
In today’s tutorial, we’ll learn how to create a simple card interface using Android Jetpack Compose. Cards are versatile UI elements that neatly organize content in a visually appealing way. Whether you’re displaying user profiles or item details, cards are an excellent choice.
Why Use Cards?
Cards are popular because they offer a clean, organized way to display information. They act like small containers, encapsulating related data in a format that’s easy to read and understand. Jetpack Compose makes creating cards a breeze.
Create a Card in Jetpack Compose
To create a card in Jetpack Compose, we’ll be using the @Composable
function. Take a look at the example code below:
@Composable
fun Example() {
Card(
modifier = Modifier
.padding(16.dp)
.fillMaxWidth()
) {
Column(
modifier = Modifier
.fillMaxWidth()
.padding(16.dp)
) {
Text(text = "A simple Card")
}
}
}
Code Explanation
Card(...)
: This is the main composable function for creating a card. It takes a variety of arguments, but for our example, we’re usingmodifier
to add padding and fill the width of the parent.Modifier.padding(16.dp)
: Adds padding around the card. The16.dp
specifies the amount of padding in density-independent pixels.Modifier.fillMaxWidth()
: Makes the card take up the full width of its parent container.Column(...)
: Inside the card, we use a Column composable to arrange child composables vertically.Text(text = "A simple Card")
: This places a text element within the column, displaying the string “A simple Card”.
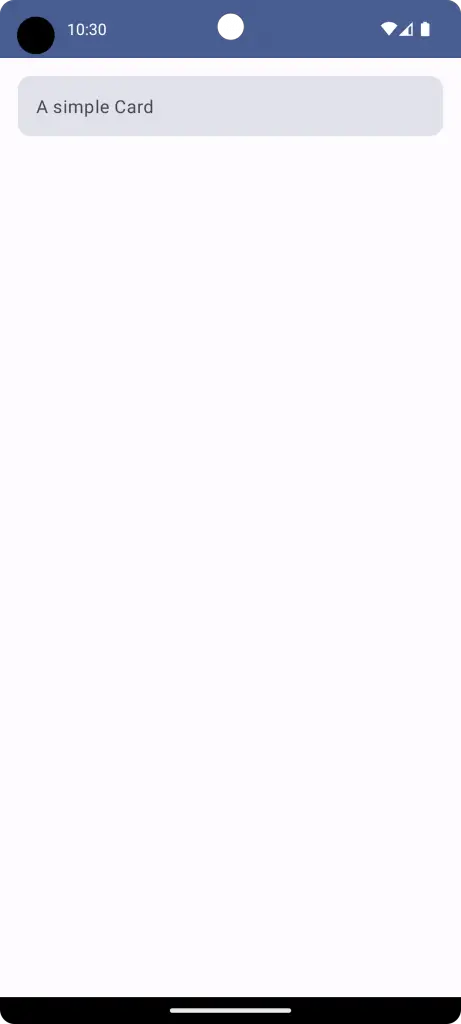
Styling and Customization
Jetpack Compose allows for easy customization. For example, you can change card background color, corner radius, and elevation to your card. The library provides various methods and properties to make your cards unique.
Creating cards in Jetpack Compose is straightforward, but the tool offers a lot of depth for those who want more control over styling and behavior. In future tutorials, we’ll dive deeper into how to make interactive and dynamic cards.
One Comment