How to Change Slider Color in Jetpack Compose
In the world of Android app development, the visual appeal of UI components plays a crucial role in enhancing user experience. Jetpack Compose, Google’s modern UI toolkit for Android, allows developers to customize UI components extensively. Among these components, the Slider stands out for its versatility.
This blog post focuses on customizing the colors of a Slider in Jetpack Compose, ensuring it aligns perfectly with your app’s design and theme.
Slider in Jetpack Compose
A Slider in Jetpack Compose is a user interface component that enables users to make selections from a range of values. Its customizable nature extends to various aspects, including its color scheme, which can significantly impact the user interface’s look and feel.
Customize the Slider Colors
Basic Slider Implementation
Let’s start with a basic Slider implementation in Jetpack Compose:
@Composable
fun MySlider() {
var sliderValue by remember { mutableStateOf(0f) }
Slider(
value = sliderValue,
onValueChange = { sliderValue = it },
valueRange = 0f..10f,
)
}
This code snippet sets up a basic slider with default colors.
Customize Slider Colors
Jetpack Compose provides the SliderDefaults.colors
function to customize the color of different parts of the Slider, including the thumb, track, and tick marks.
Changing Thumb and Track Colors
To change the thumb and track colors, you can use the following code:
@Composable
fun MySlider() {
var sliderValue by remember { mutableStateOf(0f) }
Slider(
value = sliderValue,
onValueChange = { sliderValue = it },
valueRange = 0f..10f,
colors = SliderDefaults.colors(
thumbColor = Color.Red,
activeTrackColor = Color.Blue,
inactiveTrackColor = Color.Gray
)
)
}
In this example:
thumbColor
changes the color of the slider thumb.activeTrackColor
changes the color of the track portion from the start to the thumb.inactiveTrackColor
changes the color of the track portion from the thumb to the end.
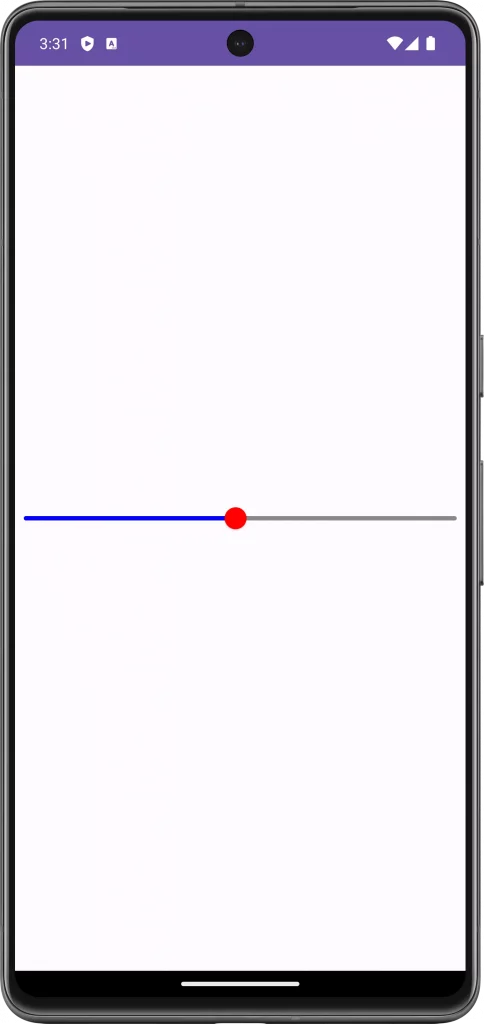
Customize Tick Marks and Overlay Color
Similarly, if your slider has tick marks or an overlay, you can customize these colors as well:
Slider(
value = sliderValue,
onValueChange = { sliderValue = it },
colors = SliderDefaults.colors(
thumbColor = Color.Red,
activeTrackColor = Color.Blue,
inactiveTrackColor = Color.Gray,
activeTickMarkColor = Color.Green,
inactiveTickMarkColor = Color.Yellow
)
)
Similarly, you can also change the colors of the slider when it is disabled using disabledActiveTrackColor, disabledActiveTickColor, disabledInactiveTrackColor and
disabledInactiveTickColor.
Customizing the colors of a slider in Jetpack Compose is a straightforward yet powerful way to enhance the visual appeal of your app. By following the steps outlined above, you can ensure that the slider not only functions well but also aligns beautifully with your app’s design.
One Comment