How to Build a Simple Counter App in Android Jetpack Compose
In this tutorial, we’ll explore the basics of state management in Android’s Jetpack Compose by creating a simple counter app. Our focus will be on using the remember
API and MutableState
to achieve real-time UI updates.
Importance of State Management
When working with dynamic content that changes over time, state management becomes crucial. For instance, our counter needs to display updated numbers each time the button is clicked. Jetpack Compose provides built-in tools to make this easy.
The Code Example
Let’s jump straight into the code. Here is the @Composable
function for our counter:
@Composable
fun Counter() {
var count by remember { mutableStateOf(0) }
Column(horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth()) {
Text(text = "The current count is $count")
Button(onClick = { count++ }) {
Text(text = "Add")
}
}
}
Code Explanation
var count by remember { mutableStateOf(0) }
: We useremember
andmutableStateOf
to manage the state of our counter. This line initializescount
to zero and keeps track of its value.Column(...)
andText(...)
: These are layout and text composables that organize our UI.Button(onClick = { count++ })
: This button, when clicked, increments the value ofcount
.
Complete Code Example
Now let’s look at the complete Kotlin code for the counter app:
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
Counter()
}
}
}
}
}
}
@Composable
fun Counter() {
var count by remember { mutableStateOf(0) }
Column(horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth()) {
Text(text = "The current count is $count")
Button(onClick = { count++ }) {
Text(text = "Add")
}
}
}
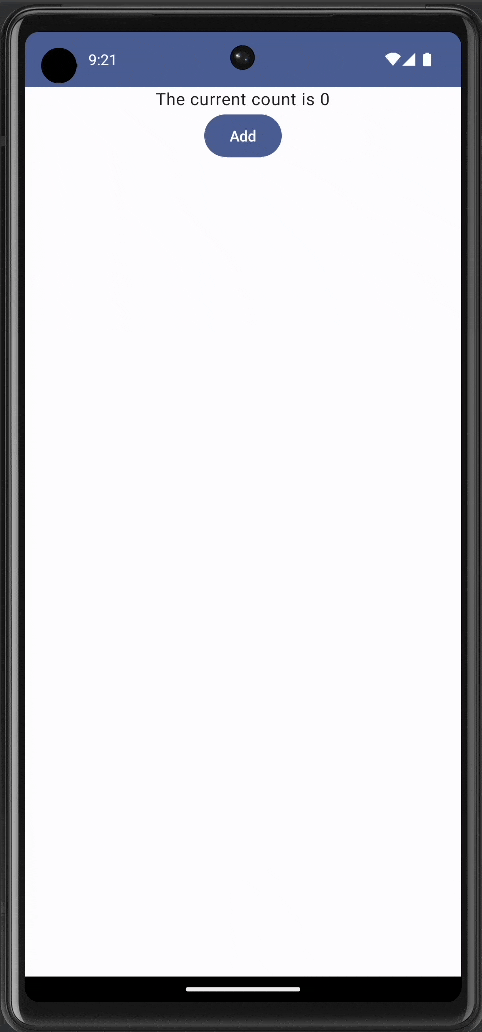
State management in Jetpack Compose is a broad topic with many features and utilities. This simple counter example should give you a good starting point. For more advanced topics in state management, consider diving into the official documentation.