How to Change Card Border Radius in Android Jetpack Compose
Among the myriad of composables offered by Jetpack Compose is the Card composable, a versatile UI element that encapsulates related content. Cards not only provide a neat way to group related content, but also offer customization options to suit different UI design needs.
In this blog post, we are going to focus on one of these customization options – adjusting the Card’s border radius.
Border Radius in Card Composable
The border radius of a Card determines how rounded the corners of the Card are. In Jetpack Compose, the Card composable provides an easy way to customize this property using the shape parameter.
How to Set Border Radius in Card
The shape parameter of the Card composable allows us to set the border radius of the card. Here’s an example:
Card(
modifier = Modifier.fillMaxWidth().padding(20.dp),
shape = RoundedCornerShape(20.dp)
) {
Text("Hello from Jetpack Compose!", Modifier.padding(16.dp))
}
In the code snippet above, we have a Card with a border radius of 20.dp. This is achieved by using the RoundedCornerShape function and passing the desired radius as a parameter. See the output given below.
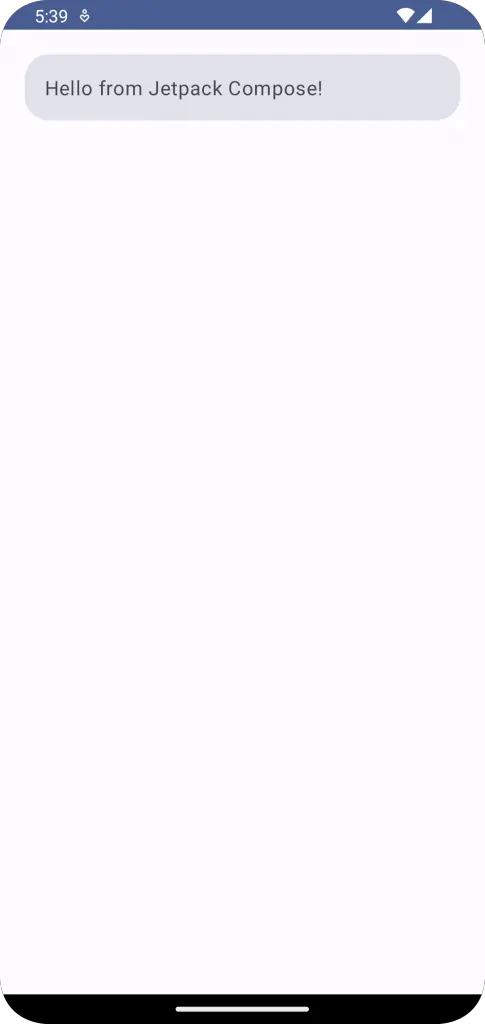
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.shape.RoundedCornerShape
import androidx.compose.material3.Card
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
CardExample()
}
}
}
}
}
@Composable
fun CardExample() {
Column {
Card(
modifier = Modifier.fillMaxWidth().padding(20.dp),
shape = RoundedCornerShape(20.dp)
) {
Text("Hello from Jetpack Compose!", Modifier.padding(16.dp))
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
CardExample()
}
}
Fine-Tuning the Border Radius
Jetpack Compose provides a high level of customization. If you want to set different radius values for each corner of the Card, you can do so by passing four parameters (one for each corner) to the RoundedCornerShape function:
Card(
shape = RoundedCornerShape(16.dp, 8.dp, 8.dp, 16.dp)
) {
Text("Hello from Jetpack Compose!", Modifier.padding(16.dp))
}
In this example, the top left and bottom right corners of the Card have a radius of 16.dp, while the top right and bottom left corners have a radius of 8.dp.
The border radius is a design tool that can greatly affect the aesthetics of your UI. Whether you need a Card with sharp corners, slightly rounded corners, or different radius for each corner, Jetpack Compose has you covered.