How to Create Password TextField in Android Jetpack Compose
Creating a secure and functional password input field is a common need in mobile app development. Jetpack Compose provides a simple yet robust way to accomplish this.
In this tutorial, we will learn how to use the TextField
composable to create a password input field. You’ll discover how to handle different keyboard options and apply visual transformations to mask the entered characters.
What is TextField Composable?
The TextField
composable in Jetpack Compose is a flexible UI element for text input. You can use it for various types of text fields, like email, password, or just plain text. It comes with several customization options to fit your specific needs.
Basic Password TextField Example
To create a simple password input field, you need to set up a few parameters in your TextField
composable. The KeyboardOptions
parameter allows you to specify the keyboard type, and the visualTransformation
parameter hides the entered text for security.
Here’s a simple example code snippet:
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput,
onValueChange = {textInput = it},
placeholder = { Text("Password") },
keyboardOptions = KeyboardOptions(keyboardType = KeyboardType.Password),
visualTransformation = PasswordVisualTransformation(),
modifier = Modifier.fillMaxWidth()
.padding(10.dp))
}
Complete Code
Below is a complete example that demonstrates how to add a password TextField in a Jetpack Compose application.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.text.KeyboardOptions
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TextField
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.text.input.KeyboardType
import androidx.compose.ui.text.input.PasswordVisualTransformation
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextFieldExample()
}
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput,
onValueChange = {textInput = it},
placeholder = { Text("Password") },
keyboardOptions = KeyboardOptions(keyboardType = KeyboardType.Password),
visualTransformation = PasswordVisualTransformation(),
modifier = Modifier.fillMaxWidth()
.padding(10.dp))
}
In this example, the TextField
composable is wrapped inside a Column
. We use the remember
function from Jetpack Compose to maintain the state of the entered text.
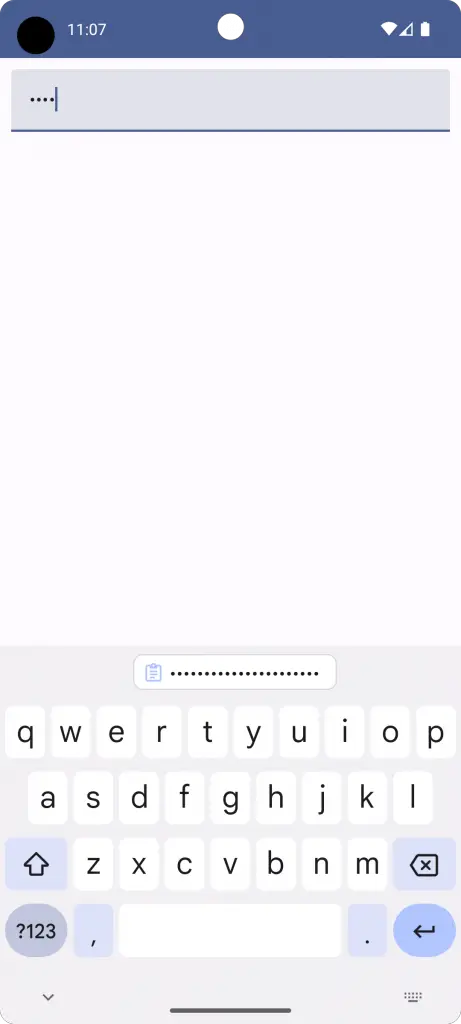
I hope this tutorial helps you understand how to create a secure password TextField using Jetpack Compose. It’s relatively straightforward but highly functional, allowing you to build secure and user-friendly mobile apps.