How to Add TextField with Border in iOS SwiftUI
Text fields are essential components in many applications, allowing users to input text. In SwiftUI, adding a border to a text field not only makes it visually appealing but also enhances user experience.
In this blog post, we’ll explore how to create and customize borders for SwiftUI TextFields using a rounded border style.
Add a Rounded Border
SwiftUI provides a straightforward way to add a rounded border to a TextField using the textFieldStyle
modifier. This makes the TextField look neat and visually consistent with many UI designs.
import SwiftUI
struct ContentView: View {
@State private var text = ""
var body: some View {
TextField("Enter text", text: $text)
.padding()
.textFieldStyle(.roundedBorder)
}
}
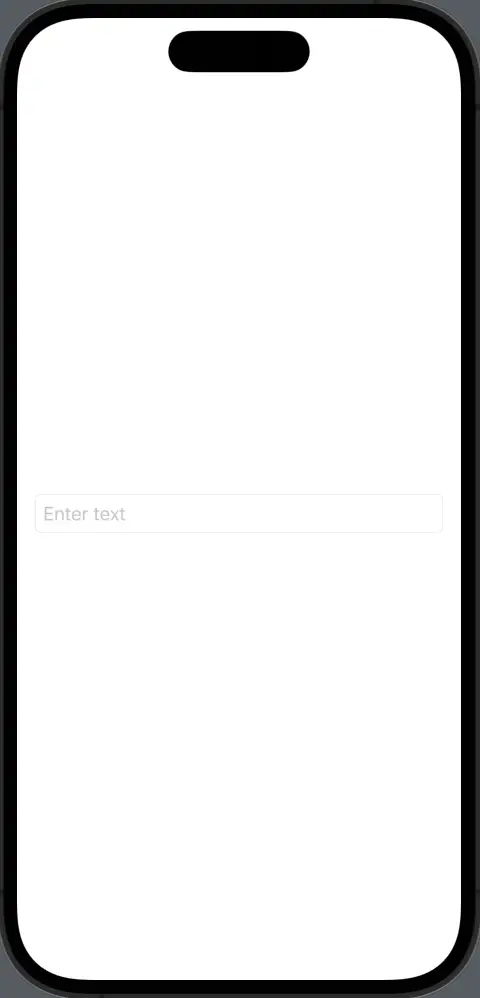
Customize Border with Overlay
For a more customized appearance, such as a specific color or line width, you can use the overlay
modifier along with rounded corners.
struct ContentView: View {
@State private var text = ""
var body: some View {
TextField("Enter text", text: $text)
.padding()
.overlay(
RoundedRectangle(cornerRadius: 10)
.stroke(Color.blue, lineWidth: 2)
)
}
}
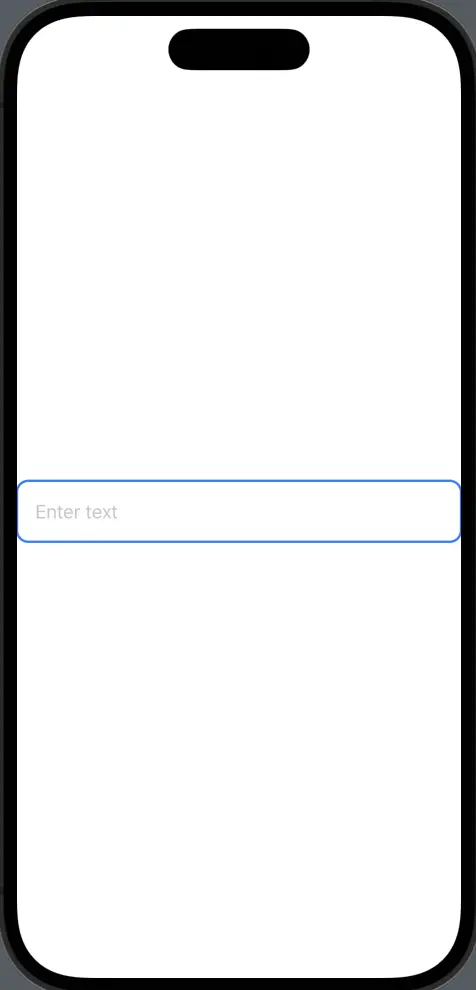
Add Padding
Padding helps to visually separate the border from the text. Using the padding
modifier, you can easily add space around the text, enhancing the visual appearance.
struct ContentView: View {
@State private var text = ""
var body: some View {
TextField("Enter text", text: $text)
.padding()
.overlay(
RoundedRectangle(cornerRadius: 10)
.stroke(Color.red, lineWidth: 2)
)
.padding()
}
}
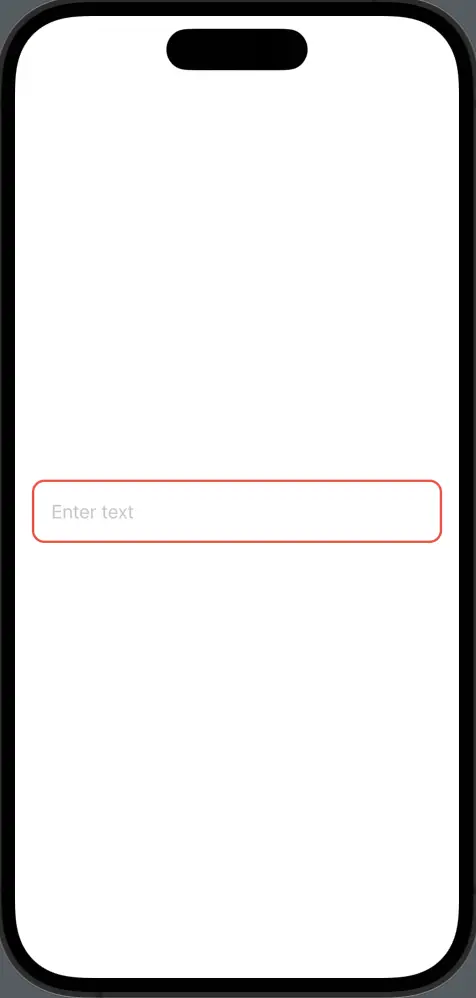
SwiftUI offers simple yet powerful ways to add and customize borders to TextFields. From using the built-in rounded border style to creating your own with overlays, you can create text fields that fit the visual theme of your app. Experiment with these techniques to give your text fields a polished and professional look.