How to Add LazyVGrid with Rounded Corner Border in SwiftUI
In SwiftUI, the LazyVGrid
component is an essential tool for creating an efficient, flexible grid that expands vertically. However, there’s more to SwiftUI’s UI toolkit than just functionality.
Adding stylistic touches like rounded corner borders can elevate your user interface. This post explores the use of LazyVGrid
with such stylized enhancements.
Add Rounded Corner Borders in SwiftUI
In SwiftUI, .overlay()
is a powerful modifier that can display an overlay view in front of or behind another view. Here, we use .overlay()
to add a border to our LazyVGrid
.
In this example, we begin with a LazyVGrid
filled with rectangles of various colors. The corners of each rectangle are rounded using the .cornerRadius(10)
modifier.
.overlay(
RoundedRectangle(cornerRadius: 10)
.stroke(Color.black, lineWidth: 4)
)
The RoundedRectangle
shape provides a rectangular shape with rounded corners, which we’re using as the overlay. We’ve added a stroke (or border) to this rounded rectangle with a width of 4 and color black.
The LazyVGrid
in SwiftUI is designed to organize child views into a grid of rows and columns. It’s “lazy” because it only loads views that are currently visible, boosting the performance of your apps.
struct ContentView: View {
let columns = [
GridItem(.flexible()),
GridItem(.flexible()),
]
let colors: [Color] = [.red, .green, .blue, .orange, .yellow, .pink, .purple, .gray]
var body: some View {
ScrollView {
LazyVGrid(columns: columns, spacing: 20) {
ForEach(colors, id: \.self) { color in
Rectangle()
.fill(color)
.frame(height: 100)
.cornerRadius(10)
}
}
.padding()
.overlay(
RoundedRectangle(cornerRadius: 10)
.stroke(Color.black, lineWidth: 4)
)
.padding()
}
}
}
We’ve added .padding()
twice in our code: once around the LazyVGrid
to provide space between the grid and the overlay, and again around the overlay to keep it from touching the edge of the screen.
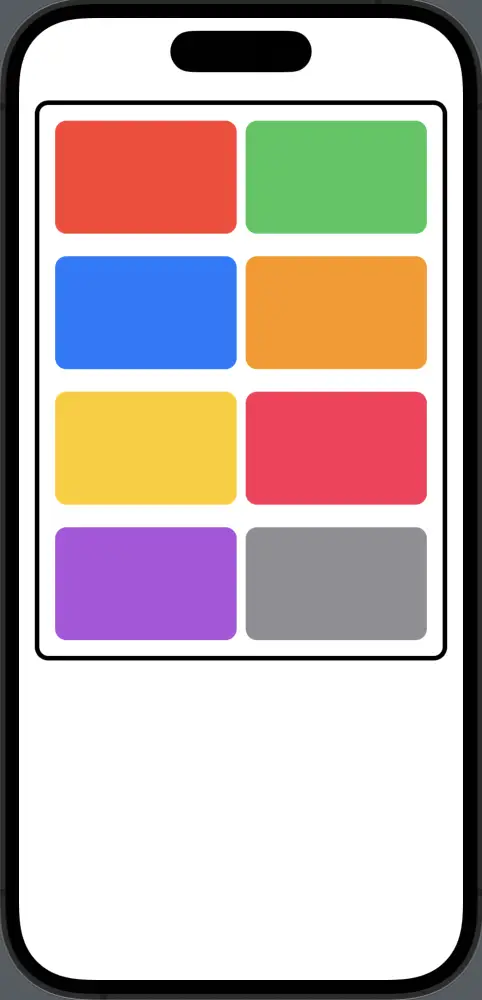
SwiftUI’s LazyVGrid
is a powerful UI tool that can create efficient, responsive layouts. By using the overlay
modifier and adding some padding, we can further enhance our grid with rounded corner borders for a polished and appealing UI.