How to Add LazyHGrid in iOS SwiftUI
If you are familiar with SwiftUI, you know it revolutionized the way we design and develop user interfaces. In this blog post, we’ll explore SwiftUI’s LazyHGrid, a highly adaptable and efficient layout tool for horizontal data presentation.
What is LazyHGrid?
LazyHGrid in SwiftUI is a container that arranges its children in a grid that grows horizontally. Its functionality is similar to that of LazyVGrid, but it extends along the horizontal axis.
The “lazy” characteristic refers to the grid only loading and rendering the items visible on screen. This leads to efficient performance, even when dealing with extensive data sets.
What is GridItem?
Before we dive into LazyHGrid, it’s crucial to understand the role of GridItem. It’s a flexible structure that describes the layout of items in a LazyHGrid or LazyVGrid. Each GridItem represents a column in a vertical grid or a row in a horizontal grid. You can define the size of GridItems using three different types: .fixed
, .flexible
, and .adaptive
.
An Example of SwiftUI LazyHGrid
Let’s take a look at an example of how to create a LazyHGrid.
struct ContentView: View {
let rows = [
GridItem(.flexible()),
GridItem(.flexible())
]
let colors: [Color] = [.red, .green, .blue, .orange, .yellow, .pink, .purple, .gray]
var body: some View {
ScrollView(.horizontal) {
LazyHGrid(rows: rows, spacing: 20) {
ForEach(colors, id: \.self) { color in
Rectangle()
.fill(color)
.frame(width: 100)
}
}
.padding()
}
}
}
The ScrollView
has a .horizontal
parameter, making it scroll horizontally. The LazyHGrid
uses the rows
array to determine its layout. Here, we’ve defined two flexible rows. The ForEach
loop iterates through the colors
array, creating a rectangle filled with each color.
This example demonstrates a basic LazyHGrid with two rows. It displays rectangles filled with different colors, scrolling horizontally.
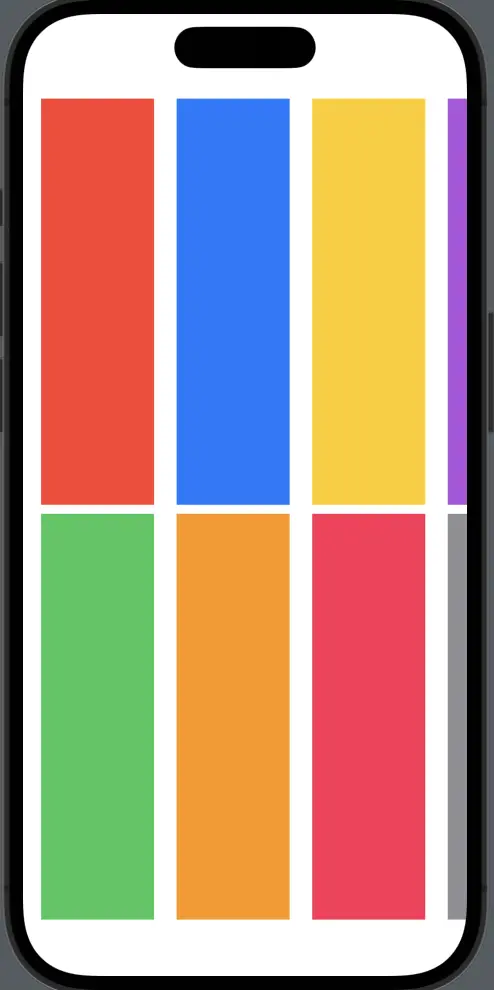
LazyHGrids are handy for instances where horizontal scrolling of content is preferred or necessary. This could be a movie app displaying various movie posters, a calendar app showing days of the week, or a gallery app showcasing images.
SwiftUI’s LazyHGrid provides an efficient way to display horizontally scrolling content. Its performance optimization and adaptability to different kinds of data make it a valuable tool in SwiftUI. Understanding and utilizing LazyHGrid effectively can significantly enhance the user interface of your SwiftUI app.
at how to use LazyHGrid effectively and efficiently.