How to Customize ProgressView Color in iOS SwiftUI
Progress indicators are essential UI elements that keep users informed about ongoing tasks. In SwiftUI, the ProgressView
is used for this purpose. While the default appearance is good enough for most cases, you might want to customize it to better match your app’s theme.
One common customization is changing the color. In this blog post, we’ll explore how to customize the color of ProgressView
in SwiftUI.
What is a ProgressView?
A ProgressView
in SwiftUI is a UI element that indicates the progress of a task. It can be either determinate, showing the exact percentage of completion, or indeterminate, showing just an animation indicating ongoing activity.
Change Color of a Determinate ProgressView
Example: Basic Determinate ProgressView
Here’s a simple example of a determinate ProgressView
.
import SwiftUI
struct ContentView: View {
@State private var progress: Double = 0.5
var body: some View {
ProgressView(value: progress, total: 1.0)
}
}
This ProgressView
shows 50% completion as the value is set to 0.5 out of a total of 1.0.
Customize the Color
To change the color, you can use the .progressViewStyle
modifier.
import SwiftUI
struct ContentView: View {
@State private var progress: Double = 0.5
var body: some View {
ProgressView(value: progress, total: 1.0).tint(.red)
}
}
The tint modifier allows you to set a tint color to the ProgressView. Here, we set it to red.
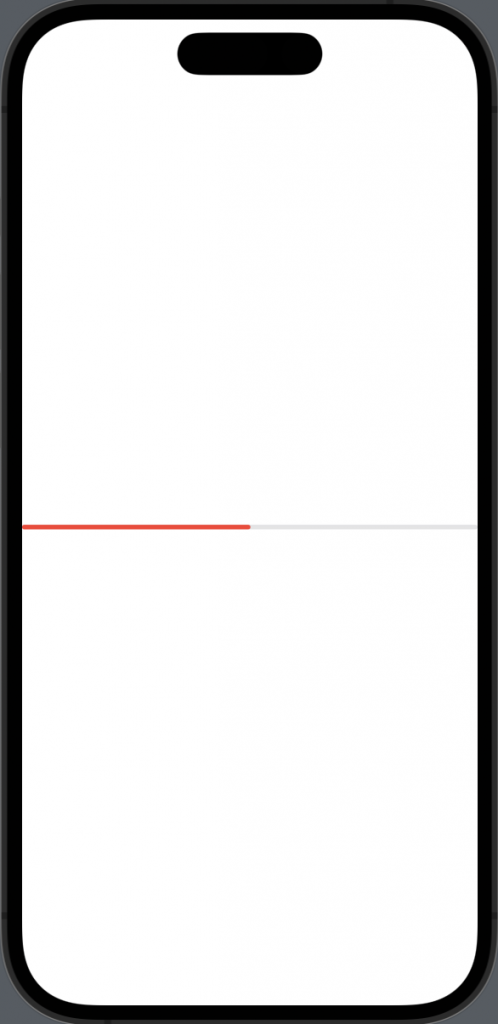
Change Color of an Indeterminate ProgressView
Example: Basic Indeterminate ProgressView
Here’s how you create a basic indeterminate ProgressView
.
ProgressView()
This creates an indeterminate ProgressView
that shows a spinning indicator.
Customize the Color
Just like with the determinate ProgressView
, you can change the color using the tint modifier.
import SwiftUI
struct ContentView: View {
var body: some View {
ProgressView().tint(.red)
}
}
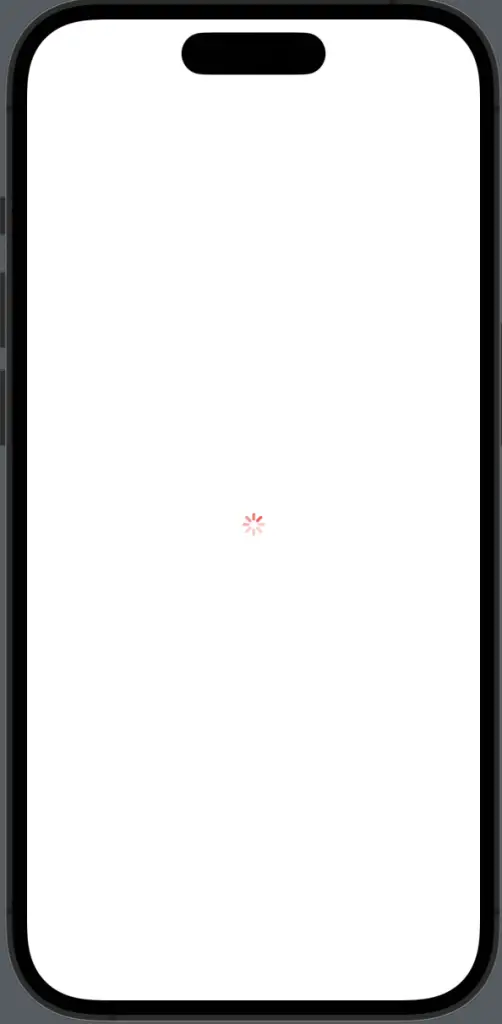
Customize Color for Different Styles
You can also change the tint color when you use a predefined ProgressView style.
import SwiftUI
struct ContentView: View {
var body: some View {
ProgressView().progressViewStyle(.circular).tint(.red)
}
}
Customizing the color of ProgressView
in SwiftUI is straightforward but offers a significant impact on the user experience. Whether you’re working with determinate or indeterminate progress indicators, SwiftUI provides easy ways to match them to your app’s overall design.