How to Implement ScrollTo with LazyVStack
SwiftUI’s ScrollView, when combined with LazyVStack, offers a seamless way to create a vertically scrolling list of items. However, navigating such lists, especially when they are large, can be a challenge. That’s where the scrollTo
function comes in.
In this blog post, we’ll show you how to leverage scrollTo
within your SwiftUI app’s LazyVStack for better user experience.
LazyVStack Overview
Before we jump into scrollTo
, it’s essential to have a grasp of LazyVStack. A LazyVStack is a SwiftUI view that arranges its child views along a vertical axis, creating them only when they’re needed. This lazy rendering offers improved performance when working with large numbers of child views.
The Need for ScrollTo
For applications where the content exceeds the screen size, scrolling is inevitable. But what if you want to programmatically control the scroll position? For instance, imagine an app that includes an alphabetical directory. You might want to provide a feature that allows users to jump directly to “M” names, skipping everything before them.
The scrollTo
function allows developers to programmatically scroll to any view within the ScrollView.
Implement ScrollTo with LazyVStack
Now let’s see how to apply scrollTo
within a ScrollView containing a LazyVStack. To do this, we’ll use an identifier to mark the target of our scroll action.
Here’s an example:
struct ContentView: View {
var body: some View {
ScrollViewReader { value in
VStack {
Button("Scroll to Bottom") {
value.scrollTo(50)
}
.padding()
ScrollView {
LazyVStack {
ForEach(1...50, id: \.self) { i in
Text("Row \(i)")
.id(i)
}
}
}
}
}
}
}
In this example, we have a button that, when pressed, will scroll to the bottom of the ScrollView (the 50th row). The scrollTo
function takes the identifier of the view we want to scroll to—in this case, the integer i
, which is attached as an ID to each Text view in the LazyVStack.
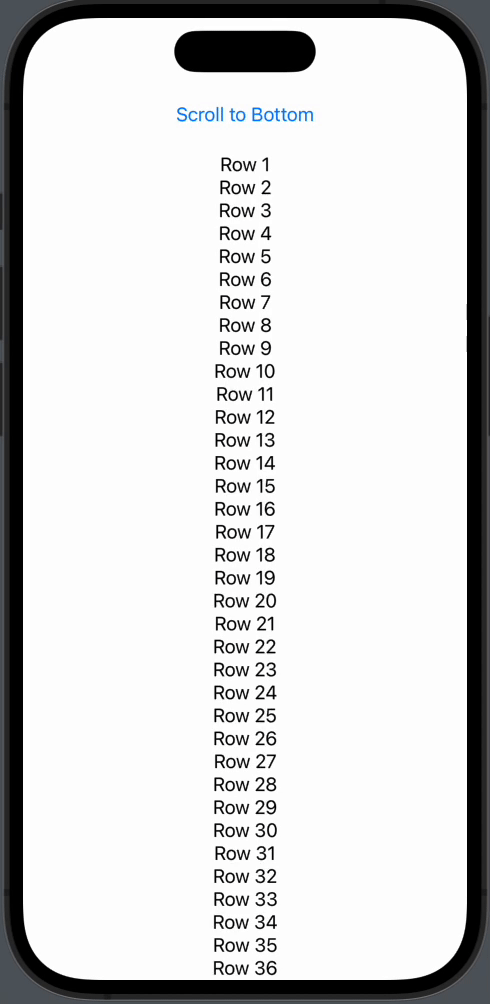
Considerations for ScrollTo
Remember that scrollTo
works with any view inside a ScrollView, not just those in LazyVStack. This makes it a versatile tool for improving navigation in your SwiftUI apps.
Also, while scrollTo
provides a useful way to control scrolling, remember to use it judiciously. Too much automatic scrolling can disorient users or make your UI feel less interactive. Always consider your users’ needs and preferences when deciding when and where to implement automatic scrolling.
The scrollTo
function adds another layer of interactivity and navigation to your SwiftUI apps. Used wisely with LazyVStack and ScrollView, it can significantly enhance your user’s experience, especially when dealing with long lists of items.