How to Set Default Value in iOS SwiftUI TextField
Providing default values for text fields can significantly improve the user experience of your app by providing hints or pre-filled information. In SwiftUI, setting a default value in a TextField is simple yet valuable for various use cases like login forms or search bars.
This blog post will guide you through setting a default value in a SwiftUI TextField.
How to Set a Default Value
In SwiftUI, you can set a default value for a TextField by initializing a state variable with the desired default value. Let’s see how it’s done:
struct ContentView: View {
@State private var text = "Default Value"
var body: some View {
TextField("Placeholder", text: $text)
.padding()
}
}
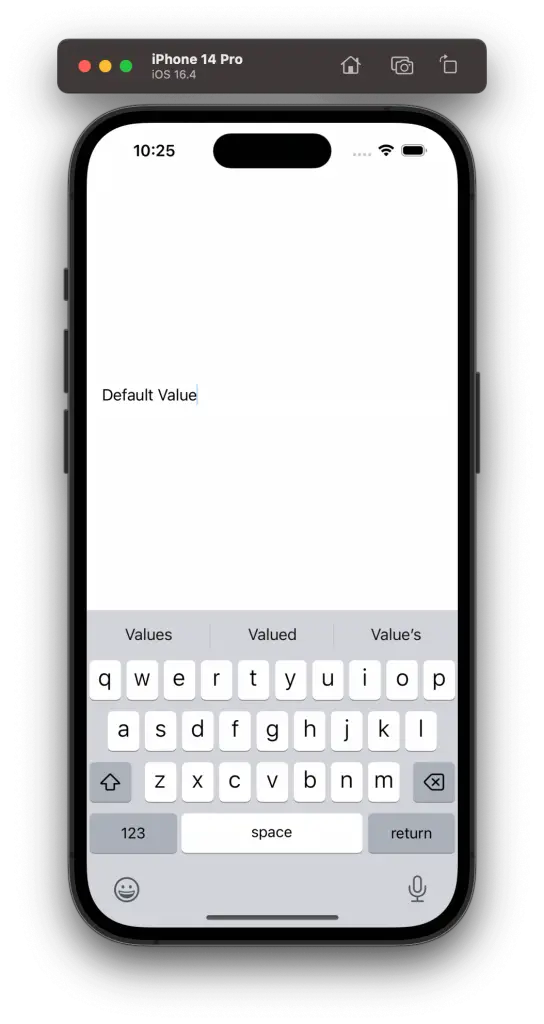
Code Explanation
@State
Property Wrapper
The @State
property wrapper is used to declare a private variable text
, initialized with the default value “Default Value”. This variable is both readable and writable, allowing the TextField to display and update the value.
TextField
The TextField is created with a placeholder "Placeholder"
and bound to the text
state variable. Since text
is initialized with a default value, the TextField will show this value when rendered.
Padding
Padding is added around the TextField to provide some space and enhance the visual appearance.
When to Use Default Values
Setting a default value in a text field can be highly beneficial in various scenarios, such as:
- Pre-filling Information: If the user has previously entered certain details, you can provide those as default values during subsequent visits.
- Providing Hints: You can use default values to give users hints about the expected input, such as a particular format for a date or phone number.
- Enhancing Usability: Default values can simplify user interaction by reducing the amount of typing required, especially in forms with common or repetitive entries.
Setting a default value in SwiftUI TextField can enhance the user experience and usability of your app. By utilizing the @State
property wrapper, you can quickly create pre-filled or hint-providing text fields. Combining this with other SwiftUI modifiers, you can create engaging and user-friendly text input interfaces.