How to Create Scrollable TextField in iOS SwiftUI
When handling user input in mobile applications, creating a scrollable text field can enhance the user experience, especially when dealing with lengthy text.
In SwiftUI, creating a scrollable TextField is simplified by using the axis
property. This blog post will explore how to make a TextField scrollable by following the given code example.
Create a Scrollable TextField
Below is the code snippet illustrating how to create a scrollable TextField in SwiftUI:
import SwiftUI
struct ContentView: View {
@State var text = ""
var body: some View {
TextField("Enter your text", text: $text, axis: .vertical)
.textFieldStyle(.roundedBorder)
.frame(height: 50)
.padding()
}
}
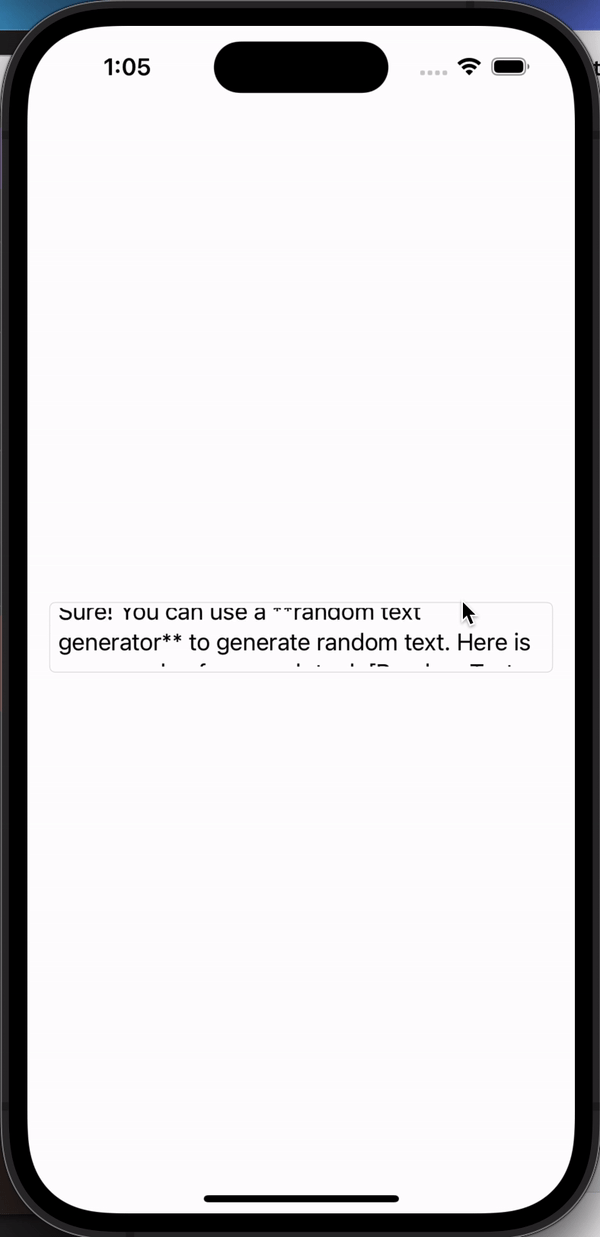
Key Components of the Code
axis
Property
The axis
property is set to .vertical
, enabling vertical scrolling within the TextField.
textFieldStyle
Modifier
The .textFieldStyle(.roundedBorder)
modifier adds a rounded border to the TextField, giving it a pleasing appearance.
frame
Modifier
The frame height of the TextField is set to 50, defining the visible area for the input.
padding
Modifier
Padding is added around the TextField to provide spacing and align the element appropriately within the view.
Advantages and Considerations
Using the axis
property simplifies the creation of a scrollable TextField. It’s a straightforward approach that allows you to achieve the desired functionality without needing complex calculations or additional views.
However, remember to consider the available space and design requirements of your app to ensure that the scrollable TextField provides an optimal user experience.
The addition of a scrollable TextField can greatly enhance the user interaction within an application, especially when dealing with large text inputs. The provided example demonstrates how to implement this feature using the axis
property, combined with other SwiftUI modifiers.
By following this approach, you can create an elegant and functional scrollable TextField that fits seamlessly into your application’s design.