How to Add HStack with Rounded Border in iOS SwiftUI
SwiftUI’s HStack allows you to layout views horizontally, forming the foundation of many user interfaces. In this blog post, we’re going to focus on enhancing these views by adding a border and adjusting the corner radius.
HStack Overview
HStack, or horizontal stack, lines up its child views along a horizontal axis. With a few simple modifiers, we can add style and polish to an HStack.
Add HStack
Adding a border to your HStack is straightforward. You just use the .border
modifier and specify the color and width you prefer.
Step 1: Create an HStack
First, create an HStack. Here’s an example with a couple of Text views.
HStack {
Text("Welcome to")
Text("SwiftUI")
}
Add HStack Border with Corner Radius
Creating rounded corners on your HStack can also be done by utilizing the overlay modifier in SwiftUI. This gives you additional control and flexibility.
Step 2: Implement Corner Radius with an Overlay
Instead of the .cornerRadius
modifier, you can use the .overlay
modifier with a RoundedRectangle shape to achieve rounded corners. Here’s how you would do it:
HStack {
Text("Welcome to")
Text("SwiftUI")
}.padding()
.overlay(
RoundedRectangle(cornerRadius: 20)
.stroke(Color.blue, lineWidth: 4)
)
In this example, we first apply padding to the HStack. We then overlay a RoundedRectangle with a corner radius of 20, and stroke it with a blue border of width 4. This results in an HStack with nicely rounded corners and a blue border.
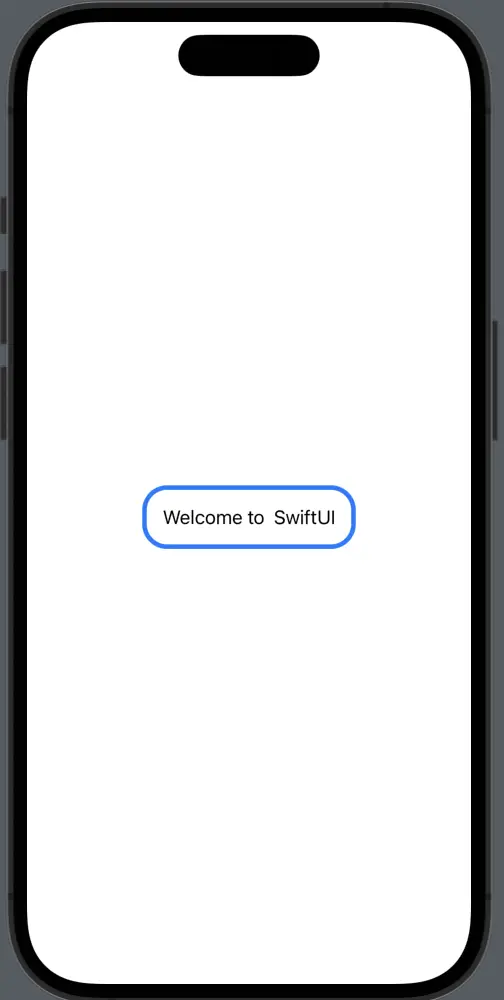
Following is the complete code for reference.
import SwiftUI
struct ContentView: View {
var body: some View {
HStack {
Text("Welcome to")
Text("SwiftUI")
}.padding()
.overlay(
RoundedRectangle(cornerRadius: 20)
.stroke(Color.blue, lineWidth: 4)
)
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
SwiftUI’s HStack not only allows for horizontal alignment of views, but also offers a multitude of ways to customize and enhance these views. Using an overlay with RoundedRectangle for creating rounded corners is a fine example of this versatility.