How to Create Translucent Background for a Sheet in SwiftUI
In SwiftUI, sheets are a versatile way to present secondary views or modals. While sheets come with a default background color, there might be scenarios where you want to make the background translucent. This can add a layer of depth to your UI and make it more visually appealing.
In this blog post, we’ll explore how to create a translucent background for a sheet in SwiftUI using the .presentationBackground
modifier and its various options.
Default Sheet Background
By default, SwiftUI sheets come with a standard background color, usually white or a system background color, depending on the device’s appearance settings (light or dark mode).
Create a Translucent Background
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
VStack{
Button("Show Sheet") {
showModal.toggle()
}
.sheet(isPresented: $showModal) {
VStack{
Text("Hello, I'm a sheet!")
Button("Dismiss"){
showModal = false
}
}.presentationDetents([.medium]).presentationBackground(.ultraThinMaterial)
}
}.frame(maxWidth: .infinity, maxHeight: .infinity).background(.yellow)
}
}
Code Explanation
.presentationBackground(.ultraThinMaterial)
: We use the.presentationBackground
modifier and set its value to.ultraThinMaterial
to make the sheet’s background translucent.
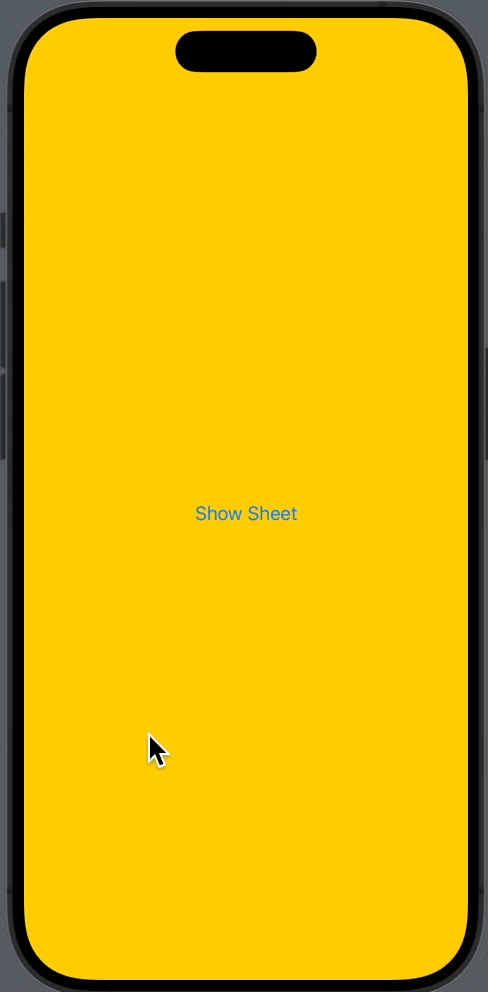
Additional Options
You can also choose other options for the background material, such as .thinMaterial
, .thickMaterial
, etc. These options provide varying degrees of translucency, allowing you to fine-tune the appearance of your sheet.
Why Use a Translucent Background?
Here are some reasons why you might want to set a translucent background for a sheet:
- Design Aesthetics: A translucent background can add a layer of depth to your UI, making it more visually appealing.
- User Experience: A translucent background can help the sheet blend in with the existing content, providing a more seamless user experience.
- Overlay Effects: You might want to overlay the sheet on top of existing content without fully obscuring it, and a translucent background can help achieve this effect.
Creating a translucent background for a sheet in SwiftUI is a straightforward task, thanks to the .presentationBackground
modifier. This simple yet powerful modifier allows you to easily customize the appearance of your sheets, making them more aligned with your app’s design and enhancing the user experience.
With options like .ultraThinMaterial
, .thinMaterial
, .thickMaterial
, etc. you can fine-tune the level of translucency to fit your specific needs.