How to Disable Sheet Animation in iOS SwiftUI
In SwiftUI, animations add a dynamic flair to the user interface, making transitions between different states smooth and visually appealing. However, there are situations where you might want to disable animations, either for performance reasons or to meet specific design requirements.
In this blog post, we’ll explore how to disable animations for a sheet in SwiftUI using the Transaction
struct and withTransaction
function.
Default Animation Behavior
By default, SwiftUI sheets come with a slide-up animation when presented and a slide-down animation when dismissed. While this provides a good user experience, you might want to disable it in certain cases.
Disable Sheet Animation
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
Button("Show Sheet") {
var transaction = Transaction()
transaction.disablesAnimations = true
withTransaction(transaction) {
showModal.toggle()
}
}
.sheet(isPresented: $showModal) {
VStack{
Text("Hello, I'm a sheet!")
Button("Dismiss"){
var transaction = Transaction()
transaction.disablesAnimations = true
withTransaction(transaction) {
showModal.toggle()
}
}
}.presentationBackground(.yellow)
}
}
}
Code Explanation
var transaction = Transaction()
: We create aTransaction
struct, which allows us to modify how changes to the view hierarchy are animated.transaction.disablesAnimations = true
: We set thedisablesAnimations
property of theTransaction
totrue
to disable animations.withTransaction(transaction)
: We use thewithTransaction
function to apply the transaction settings when toggling theshowModal
state variable.
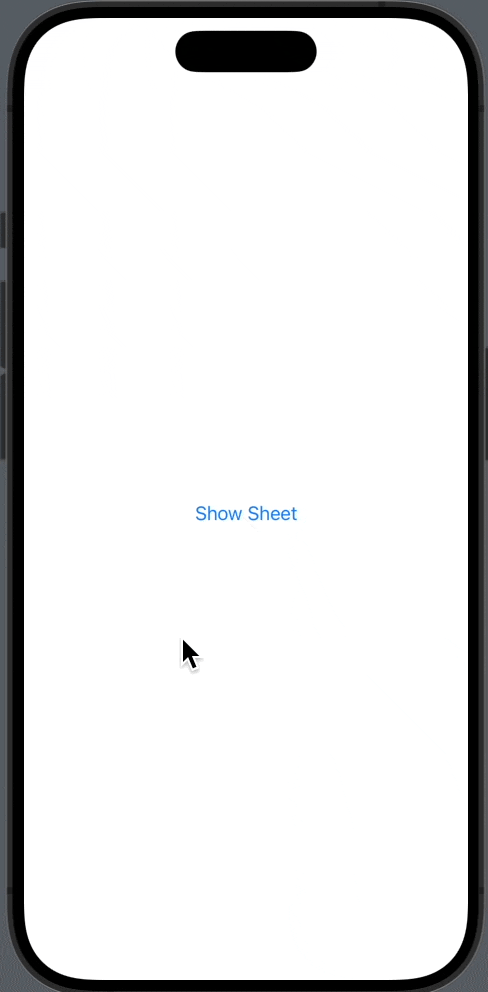
Why Disable Animations?
Here are some reasons you might want to disable animations for a sheet:
- Performance: On older devices or in complex UIs, disabling animations can improve performance.
- User Preference: Some users prefer reduced motion and may find animations distracting or uncomfortable.
- Design Requirements: Your app’s design guidelines might call for a more immediate transition without animations.
Disabling animations for a sheet in SwiftUI is straightforward, thanks to the Transaction
struct and withTransaction
function. These tools provide a simple yet effective way to control how your sheet behaves, allowing you to customize the user experience as needed.