SwiftUI: LazyHStack vs HStack
SwiftUI is a UI toolkit that comes packed with several powerful views to create flexible and dynamic interfaces. Among them, two views often used for horizontal arrangement are LazyHStack and HStack.
While they may look similar, there are key differences. In this blog post, we’ll explore these differences and provide clear examples.
What is HStack?
HStack is a SwiftUI view that arranges its children horizontally, aligning them in a row. It’s one of the basic building blocks in SwiftUI and offers an easy way to create horizontal layouts.
HStack Example
Let’s look at a simple example of HStack:
struct ContentView: View {
var body: some View {
HStack {
Text("First")
Text("Second")
Text("Third")
}
}
}
Here, the three texts are arranged horizontally within an HStack, creating a simple row.
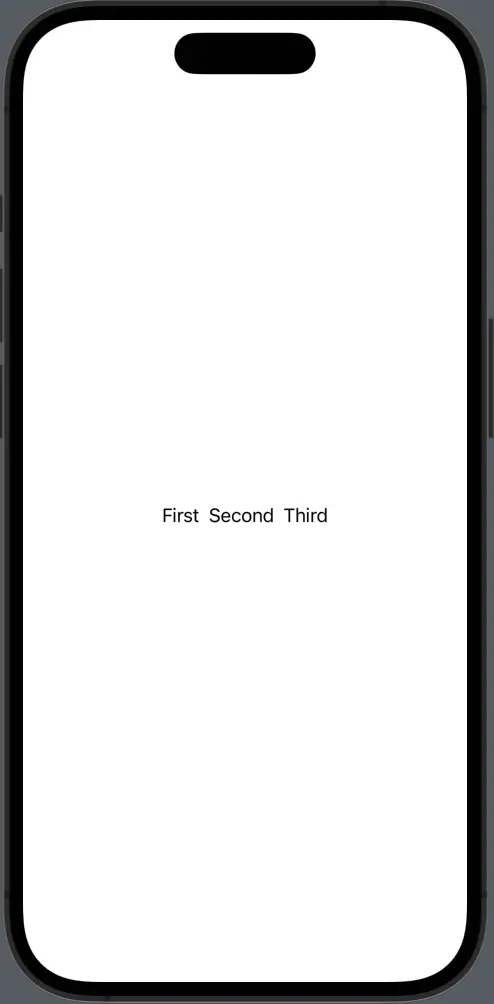
What is LazyHStack?
LazyHStack is similar to HStack but comes with a “lazy” rendering behavior. It only creates the views that are currently visible on screen. This lazy rendering can be a significant advantage, especially when dealing with a large number of views.
LazyHStack Example
Here’s an example of how to use LazyHStack:
struct ContentView: View {
var body: some View {
ScrollView(.horizontal) {
LazyHStack {
ForEach(1...1000, id: \.self) { number in
Text("\(number)")
}
}
}
}
}
This example places 1000 text views inside a LazyHStack within a horizontal ScrollView. The views are only created and rendered as they become visible while scrolling.
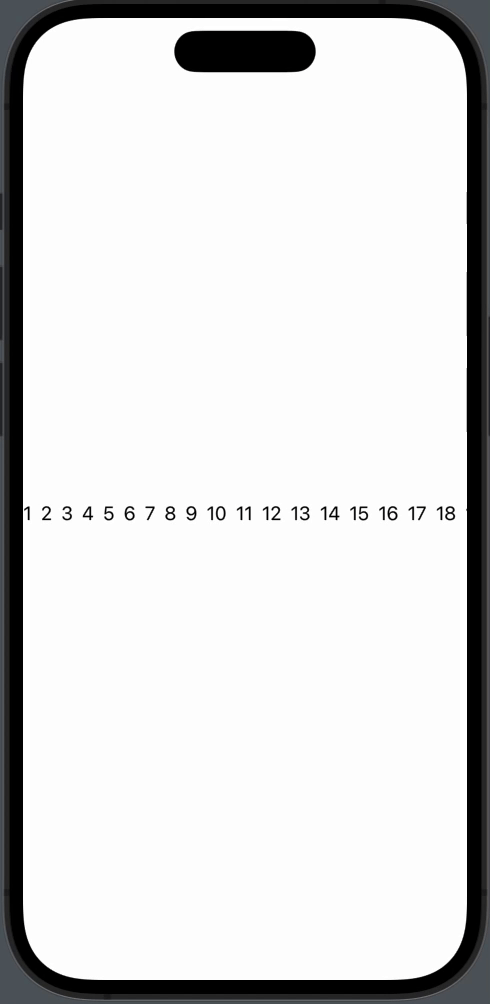
Key Differences Between LazyHStack and HStack
Rendering Behavior:
- HStack: Renders all child views at once, which might lead to performance issues when there are too many views.
- LazyHStack: Only renders the views that are visible, greatly optimizing performance with large data sets.
Usage Scenarios:
- HStack: Best for simple horizontal alignments where the number of child views is minimal and known.
- LazyHStack: Ideal for horizontal scrolling scenarios with a large number of views, like a long list of items.
Scrolling Behavior:
- HStack: Usually used without scrolling, or within a ScrollView for manual scrolling implementation.
- LazyHStack: Often used with ScrollView to achieve efficient scrolling with large content.
LazyHStack and HStack in SwiftUI serve similar purposes but are optimized for different scenarios. Use HStack for simple horizontal layouts with a limited number of views.
Opt for LazyHStack when dealing with a more significant number of views that require smooth scrolling. Understanding their differences and utilizing them appropriately can make your app more responsive and visually appealing.