How to Manage LazyHStack Spacing in iOS SwiftUI
SwiftUI has transformed the way we design user interfaces in iOS, and one of its intriguing components is the LazyHStack. Particularly focusing on the ‘spacing’ aspect of LazyHStack, this blog post will guide you through its utility, examples, and common practices.
SwiftUI LazyHStack
LazyHStack is a container view that arranges its children in a horizontal line and lazily creates views as they become visible. This helps improve the performance of your app, particularly when handling a large collection of subviews.
Importance of Spacing
The ‘spacing’ attribute is vital to provide a visually appealing layout by defining the distance between the elements in the stack. It ensures that the components aren’t cramped together, giving a more accessible and aesthetic appearance.
Usage of Spacing in LazyHStack
Spacing in LazyHStack can be controlled through the spacing
parameter. You can set it to a specific value, or leave it as nil for the default spacing.
Example with Specific Spacing
Here’s an example of a LazyHStack with specified spacing of 20 points:
struct ContentView: View {
var body: some View {
ScrollView(.horizontal) {
LazyHStack(spacing: 20) {
ForEach(1...50, id: \.self) { index in
Text("\(index)")
.frame(width: 50, height: 50)
.background(Color.green)
.cornerRadius(10)
}
}
}
}
}
In this example, the LazyHStack arranges the numbers 1 through 50 in a horizontal line, with a spacing of 20 points between each item.
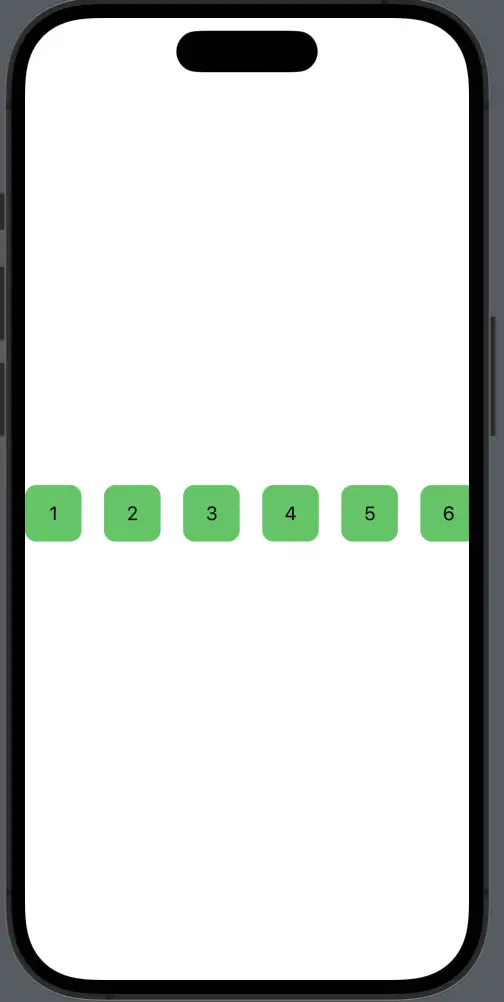
Example with Default Spacing
If you do not specify the spacing, SwiftUI will use the default value. Here’s an example:
struct ContentView: View {
var body: some View {
ScrollView(.horizontal) {
LazyHStack {
ForEach(1...50, id: \.self) { index in
Text("\(index)")
.frame(width: 50, height: 50)
.background(Color.blue)
.cornerRadius(10)
}
}
}
}
}
Without specifying the ‘spacing’ attribute, the LazyHStack applies the default spacing, resulting in a pleasing layout without any extra configuration.
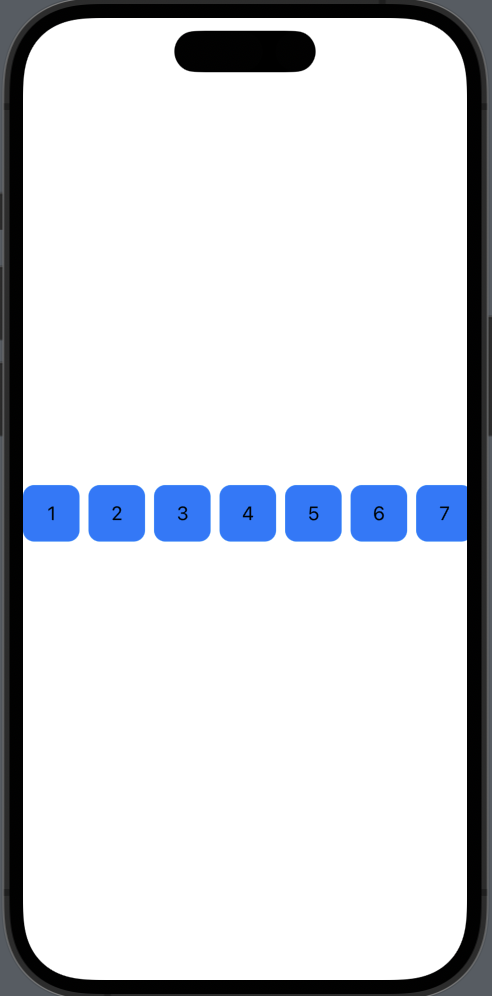
Common Practices and Considerations
- Performance Consideration: Utilizing LazyHStack with proper spacing helps create a smooth scrolling experience without compromising performance.
- Accessibility: Adequate spacing ensures that the UI remains user-friendly, providing a better experience, especially for users with accessibility needs.
- Responsiveness: Experimenting with different spacing values can help you find the perfect balance for various screen sizes and orientations.
The LazyHStack, coupled with the ‘spacing’ attribute in SwiftUI, opens up a wide range of possibilities for creating attractive and performance-optimized horizontal layouts.
Whether you’re designing a gallery, a scrolling menu, or any other horizontal collection, understanding and utilizing the ‘spacing’ parameter is essential to crafting a clean and intuitive user experience.