SwiftUI FullScreenCover Fade In and Fade Out
One of the exciting aspects of SwiftUI is its robust animation capabilities. You can create smooth transitions between different views or when presenting modals. In this post, we’ll dive into how you can achieve a fade-in and fade-out animation when working with SwiftUI’s fullScreenCover
.
What’s a FullScreenCover?
In SwiftUI, fullScreenCover
allows you to present a new view covering the entire screen. By default, it comes with a built-in slide-up animation. But what if you want to customize this behavior?
Fade-In and Fade-Out: The Code Example
Before going into the explanation, here’s the code sample we’ll be dissecting. This example will focus on creating a fade-in and fade-out animation for fullScreenCover
:
import SwiftUI
struct ContentView: View {
@State var isFullScreenCoverPresented = false
@State var isFullScreenViewVisible = false
var body: some View {
VStack {
Button("Show Full-Screen Modal") {
isFullScreenCoverPresented = true
}
}.fullScreenCover(isPresented: $isFullScreenCoverPresented) {
Group {
if isFullScreenViewVisible {
VStack {
Button("Dismiss") {
isFullScreenViewVisible = false
}
.frame(maxWidth: .infinity, maxHeight: .infinity)
.background(.yellow)
}
.onDisappear {
isFullScreenCoverPresented = false
}
}
}
.onAppear {
isFullScreenViewVisible = true
}
}
.transaction({ transaction in
transaction.disablesAnimations = true
transaction.animation = .linear(duration: 0.5)
})
}
}
Code Explanation
Let’s break down the key components of the code.
State Variables
Two @State
variables are used:
isFullScreenCoverPresented
: This controls the presentation of the full-screen modal.isFullScreenViewVisible
: Used for enabling or disabling the view visibility inside the full-screen cover.
Present the FullScreenCover
We use a Button
to toggle the isFullScreenCoverPresented
variable, which triggers the modal presentation.
Button("Show Full-Screen Modal") {
isFullScreenCoverPresented = true
}
Disable the Default Animation
The transaction
method is used to control the animations. In this example, we disable the default animations and apply a linear animation with a duration of 0.5 seconds.
.transaction({ transaction in
transaction.disablesAnimations = true
transaction.animation = .linear(duration: 0.5)
})
Fade In and Fade Out
The fade-in effect occurs when the modal appears, and the fade-out effect happens when the modal is dismissed. This is controlled by toggling isFullScreenViewVisible
.
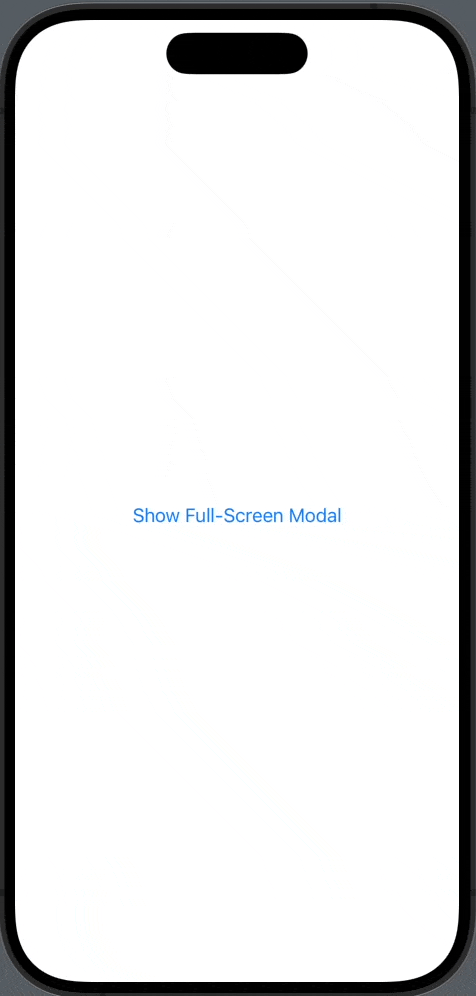
By leveraging SwiftUI’s animation capabilities and understanding the lifecycle methods, you can achieve a customized fade-in and fade-out animation for your fullScreenCover
. This provides a smoother user experience and allows you to stand out with your UI/UX design skills.