How to Disable Text Suggestions in iOS SwiftUI TextField
Text suggestions can be useful in many scenarios, but sometimes, you may want to turn off this feature for various reasons, such as privacy or specific user experience needs.
In SwiftUI, this can be achieved with a combination of modifiers. In this blog post, we’ll explore how to disable text suggestions in a SwiftUI TextField.
Code Example to Disable Text Suggestions
Below is the example code to disable text suggestions:
struct ContentView: View {
@State private var text = ""
var body: some View {
VStack {
TextField("Enter text without suggestions", text: $text)
.keyboardType(.asciiCapable)
.autocorrectionDisabled(true)
}
}
}
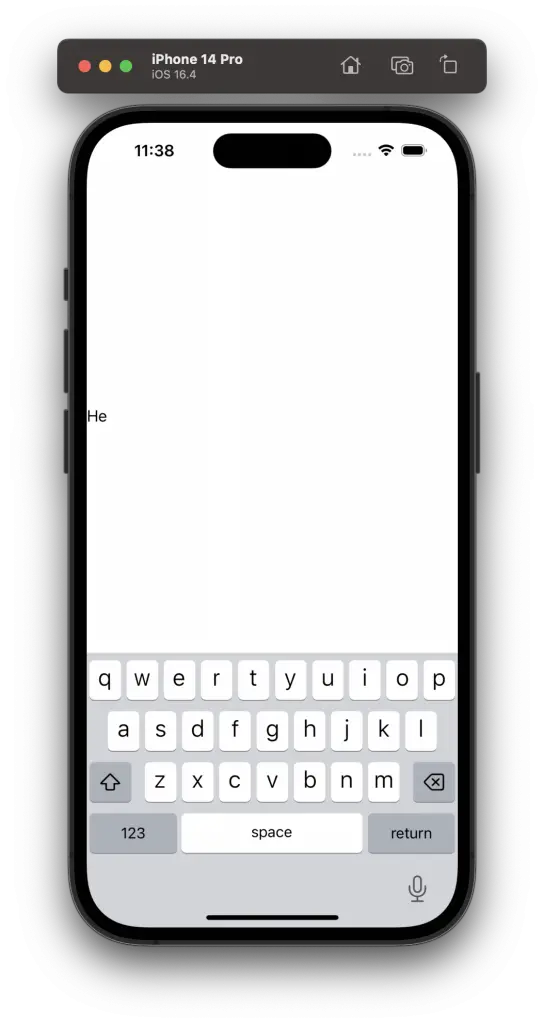
Understanding the Code
@State
Property
Using the @State
property wrapper, the text entered by the user is stored, allowing the view to react to changes.
TextField
This is where the user will input the text.
.keyboardType(.asciiCapable)
By setting the keyboard type to .asciiCapable
, we limit the keyboard to ASCII characters, which helps in disabling suggestions.
.autocorrectionDisabled(true)
This modifier is used to explicitly turn off autocorrection, further ensuring that text suggestions are disabled.
Scenarios to Disable Text Suggestions
Disabling text suggestions can be beneficial in:
- Password Fields: To protect sensitive information.
- Names or Specific Terms: When the suggestions might not apply.
- Controlled User Input: Where you want the user to input specific information without guidance.
Disabling text suggestions in SwiftUI is attainable with the combination of .keyboardType(.asciiCapable)
and .autocorrectionDisabled(true)
modifiers.
This guide provides you with the example code and explanation to implement this feature in your SwiftUI app. By understanding when and how to use these modifiers, you can create a more tailored text input experience for your users.