How to Align HStack Views at the Bottom in iOS SwiftUI
Alignment in SwiftUI plays a significant role in presenting a clean and intuitive user interface. HStack, an integral SwiftUI component, lays out views horizontally. This post delves into aligning views at the bottom within an HStack.
HStack Overview
HStack allows you to place views side-by-side in a horizontal line. The challenge arises when aligning these views, especially if they vary in size. SwiftUI, though, provides a solution: the alignment
parameter.
Align Views at the Bottom in HStack
To align views at the bottom in HStack, you should utilize the alignment
parameter in HStack and set it to .bottom
.
Step 1: Create an HStack
To begin with, create an HStack. Add a couple of views with differing heights for illustration.
HStack {
Text("First Text")
Text("Second Text").font(.largeTitle)
}
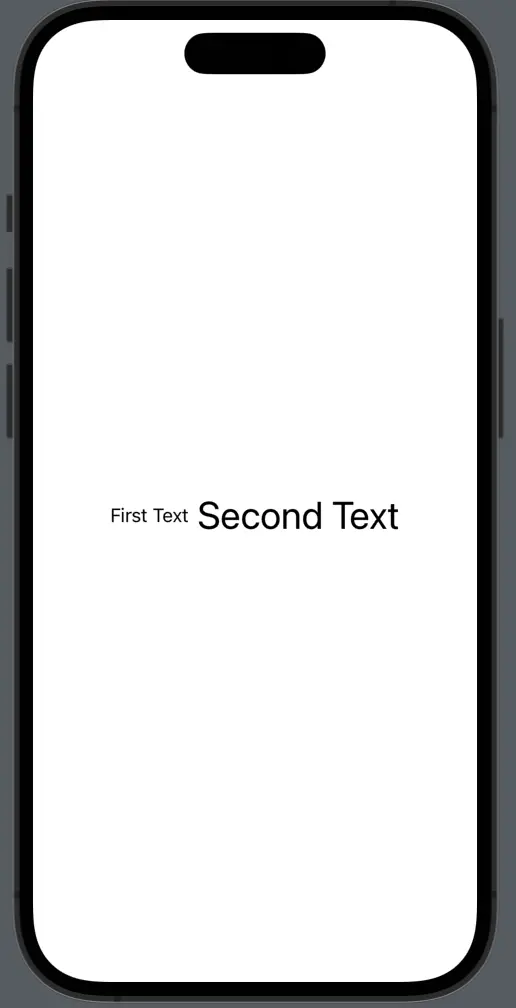
Step 2: Use the Alignment Parameter
Apply the alignment parameter to the HStack and set its value to .bottom
.
HStack(alignment: .bottom) {
Text("First Text")
Text("Second Text").font(.largeTitle)
}
This aligns the views at the bottom, despite the varying heights. SwiftUI’s alignment
parameter makes the alignment process intuitive and effortless.
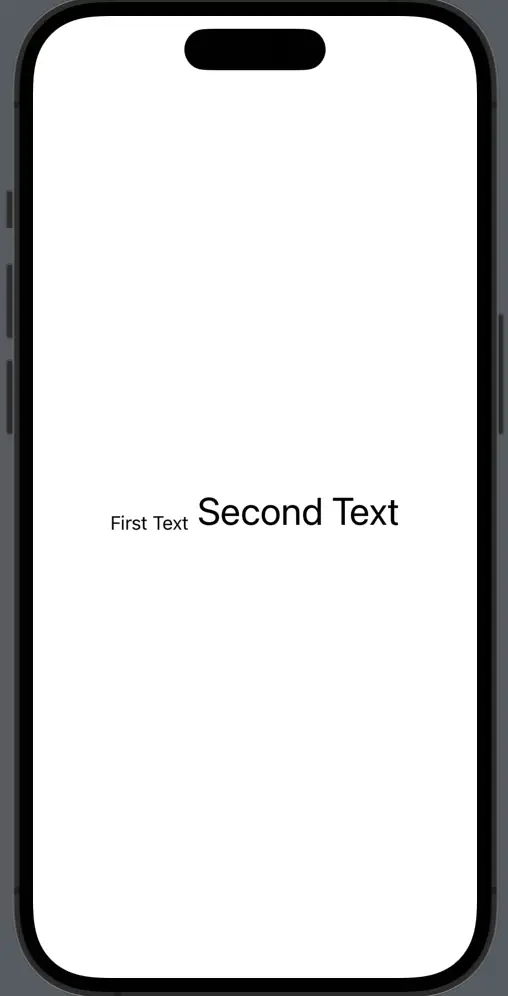
Following is the complete code for reference.
import SwiftUI
struct ContentView: View {
var body: some View {
HStack(alignment: .bottom) {
Text("First Text")
Text("Second Text").font(.largeTitle)
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Understanding how to align views within an HStack is essential in SwiftUI. By using the alignment
parameter, you can align your views at the bottom with ease, offering a better user experience and making your UI design more efficient and appealing.