How to Show and Hide ProgressView in iOS SwiftUI
In many apps, progress indicators are only needed during specific tasks, such as loading a page or downloading a file. Therefore, it’s important to know how to show and hide them appropriately.
In this blog post, we’ll explore how to control the visibility of ProgressView
in SwiftUI, complete with examples and best practices.
What is a ProgressView?
A ProgressView
in SwiftUI is a UI element that displays the progress of an ongoing task. It can be either determinate, showing a specific percentage of completion, or indeterminate, displaying a general waiting indicator.
Use State Variables
Example: Toggle ProgressView with a Button
The simplest way to control the visibility of a ProgressView
is by using a state variable. Here’s an example:
import SwiftUI
struct ContentView: View {
@State private var showProgress = false
var body: some View {
VStack {
if showProgress {
ProgressView("Loading...")
}
Button("Toggle ProgressView") {
showProgress.toggle()
}
}
}
}
We use a state variable showProgress
to control the visibility of the ProgressView
. When the button is clicked, showProgress
toggles, thereby showing or hiding the ProgressView
.
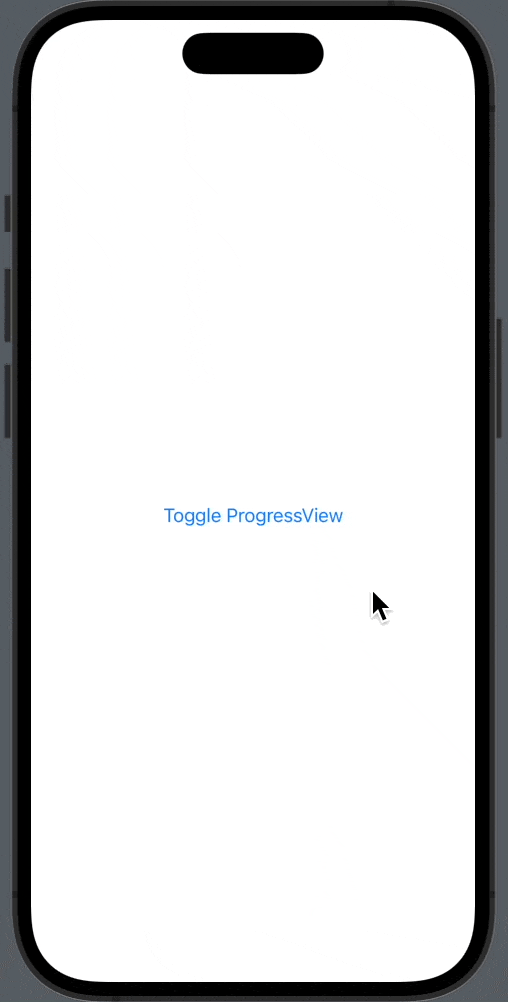
Use Conditional Logic
Example: Show ProgressView During a Task
You can also use conditional logic to show the ProgressView
only during specific tasks.
import SwiftUI
struct ContentView: View {
@State private var isLoading = false
var body: some View {
VStack {
if isLoading {
ProgressView("Downloading...")
}
Button("Start Download") {
isLoading = true
// Simulate a download task
DispatchQueue.global().async {
sleep(2)
DispatchQueue.main.async {
isLoading = false
}
}
}
}
}
}
In this example, the ProgressView
is displayed when the isLoading
state variable is true. We simulate a download task that sets isLoading
to false after 2 seconds, hiding the ProgressView
.
Use Animations
Example: Fade In and Out
You can add animations to make the transition smoother when showing or hiding the ProgressView
.
import SwiftUI
struct ContentView: View {
@State private var showProgress = false
var body: some View {
VStack {
if showProgress {
ProgressView("Loading...")
.transition(.opacity)
}
Button("Toggle ProgressView") {
withAnimation {
showProgress.toggle()
}
}
}
}
}
We use the .transition(.opacity)
modifier to add a fade-in and fade-out effect. The withAnimation
function ensures that the transition is animated.
Controlling the visibility of a ProgressView
in SwiftUI is straightforward and can be done using state variables, conditional logic, and animations. Whether you want to toggle the ProgressView
with a button or show it only during specific tasks, SwiftUI provides the flexibility to do it all.