How to Change Sheet Background Color in iOS SwiftUI
In SwiftUI, sheets are commonly used to present secondary views or modals. While they come with a default style, you may want to customize them to fit your app’s design better. One such customization is changing the background color of the sheet.
In this blog post, we’ll explore how to change the background color of a sheet in SwiftUI using the .background modifier.
Default Sheet Behavior
By default, the SwiftUI sheet comes with a standard background color, usually white or a system background color, depending on the device’s appearance settings (light or dark mode).
Change Sheet Background Color
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
Button("Show Modal") {
showModal.toggle()
}
.sheet(isPresented: $showModal) {
VStack{
Text("Hello, I'm a modal!")
Button("Dismiss") {
showModal = false
}
}.presentationDetents([.medium])
.frame(maxWidth: .infinity, maxHeight: .infinity)
.background(.yellow)
}
}
}
Code Explanation
.frame(maxWidth: .infinity, maxHeight: .infinity)
: We set the frame of the sheet to take up the maximum available width and height. This ensures that the background color will fill the entire sheet..background(.yellow)
: We use the.background
modifier to set the background color of the sheet to yellow.
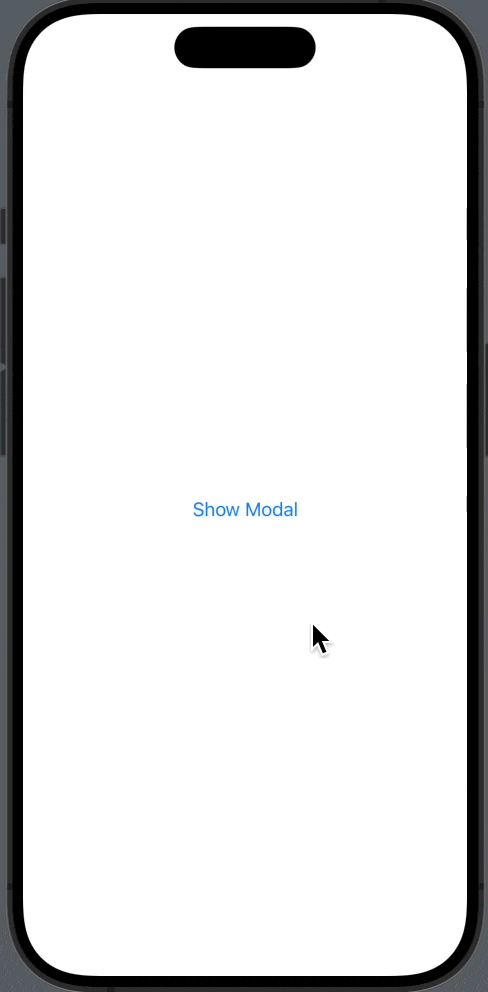
Why Change the Background Color?
Here are some reasons why you might want to change the background color of a sheet:
- Branding: To maintain brand consistency, you might want the sheet’s background color to match your app’s color scheme.
- User Experience: A different background color can help the sheet stand out, making it more noticeable and easier to interact with.
- Design Aesthetics: A custom background color can enhance the visual appeal of the sheet, making it more engaging for the user.
Changing the background color of a sheet in SwiftUI is a straightforward task thanks to the .background
modifier. This simple yet powerful modifier allows you to easily customize the appearance of your sheets, making them more aligned with your app’s design and enhancing the user experience.