How to Show Modal Using Sheet in iOS SwiftUI
Modal views are an essential part of iOS app development, allowing you to present additional information or actions without navigating away from the current screen. In SwiftUI, the Sheet modifier makes it incredibly easy to present modals.
In this blog post, we’ll explore how to show a modal using the sheet modifier in SwiftUI.
What is a Modal?
A modal is a secondary view that appears on top of the main view, usually for tasks like data input, displaying additional information, or presenting a set of actions. Modals are meant to focus the user’s attention on a single task or piece of information.
Basic Modal Presentation
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
Button("Show Modal") {
showModal.toggle()
}
.sheet(isPresented: $showModal) {
Text("Hello, I'm a modal!")
Button("Dismiss") {
showModal = false
}
}
}
}
Code Explanation
@State private var showModal = false
: We declare a state variable to control the visibility of the modal.Button("Show Modal")
: This button will trigger the modal to appear..sheet(isPresented: $showModal)
: Thesheet
modifier takes a binding to a Boolean that controls whether the sheet is presented.Button("Dismiss")
: Inside the modal, we add a button to dismiss it.showModal = false
: We set theshowModal
state variable tofalse
to dismiss the modal.
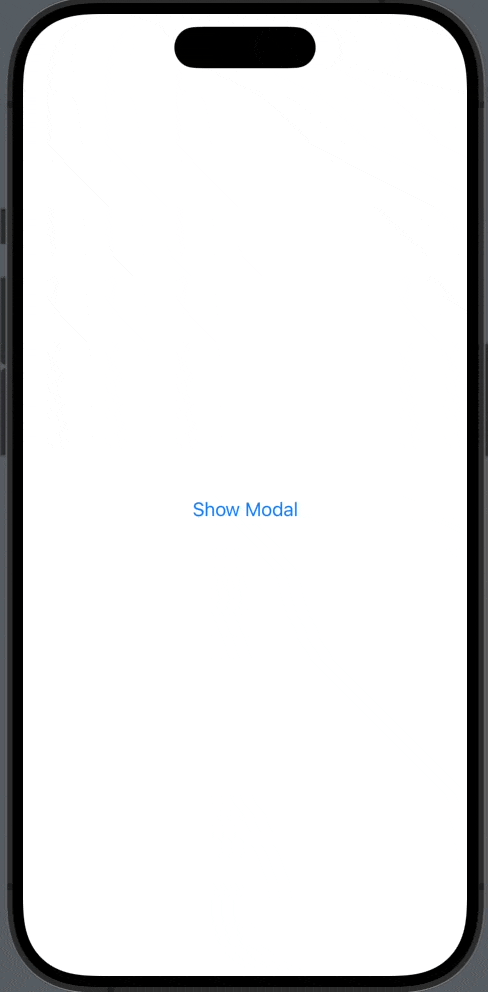
Pass Data to Modal
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
let message = "Hello from ContentView"
var body: some View {
Button("Show Modal") {
showModal.toggle()
}
.sheet(isPresented: $showModal) {
ModalView(message: message, showModal: $showModal)
}
}
}
struct ModalView: View {
let message: String
@Binding var showModal: Bool
var body: some View {
Text(message)
Button("Dismiss") {
showModal = false
}
}
}
Code Explanation
In ModalView
:
@Binding var showModal: Bool
: We have a@Binding
variable calledshowModal
. This allowsModalView
to modify theshowModal
state variable inContentView
.
In ContentView
:
.sheet(isPresented: $showModal)
: Thissheet
modifier presents the modal.ModalView(message: message, showModal: $showModal)
: While initializingModalView
, we pass in theshowModal
state variable as a binding. This allowsModalView
to modifyshowModal
and thus control its own presentation state.
By making these changes, you enable the ModalView
to dismiss itself by setting the showModal
state variable to false
. This is made possible through the use of @Binding
, which creates a two-way data binding between ContentView
and ModalView
.
If you want a full-screen modal then you should choose the fullScreenCover modifier.
Presenting modals in SwiftUI is a straightforward task thanks to the sheet
modifier. Whether you’re displaying simple text or passing data between views, the sheet
provides a simple and effective way to manage modals in your SwiftUI app.
4 Comments