How to Customize Sheet Corner Radius in iOS SwiftUI
In SwiftUI, sheets are a popular way to present secondary views or modals. While they come with a default style, you may want to customize them to fit your app’s design better. One such customization is changing the corner radius of the sheet.
In this blog post, we’ll explore how to customize the corner radius of a sheet in SwiftUI using the .presentationCornerRadius
modifier.
Default Sheet Behavior
By default, SwiftUI sheets come with a standard corner radius, which is usually rounded to a small extent. However, you might want to change this to better align with your app’s design.
Customize Sheet Corner Radius
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
Button("Show Modal") {
showModal.toggle()
}
.sheet(isPresented: $showModal) {
VStack{
Text("Hello, I'm a modal!")
Button("Dismiss") {
showModal = false
}
}.presentationDetents([.medium])
.frame(maxWidth: .infinity, maxHeight: .infinity)
.background(.yellow)
.presentationCornerRadius(20)
}
}
}
Code Explanation
.presentationCornerRadius(20)
: We use the.presentationCornerRadius
modifier to set the corner radius of the sheet to 20 points..frame(maxWidth: .infinity, maxHeight: .infinity)
: We set the frame of the sheet to take up the maximum available width and height. This ensures that the background color and corner radius will apply to the entire sheet..background(.yellow)
: We also set the background color of the sheet to yellow for demonstration purposes.
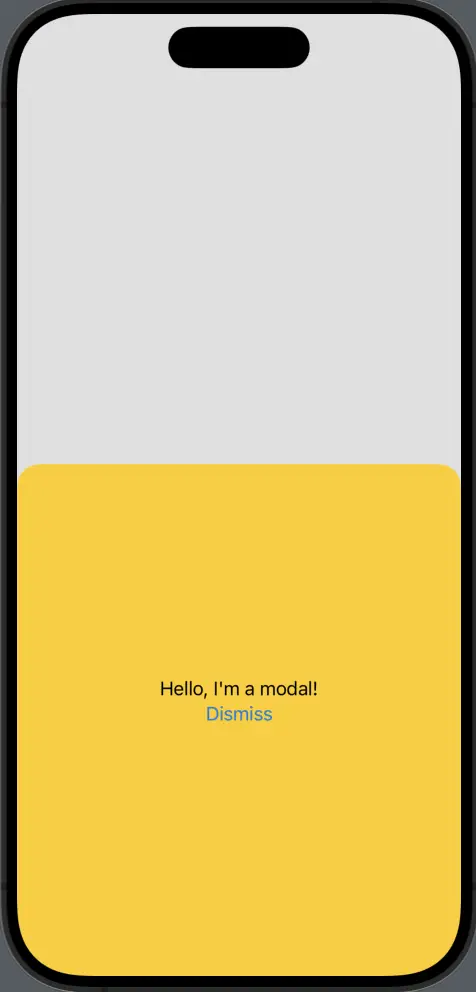
Why Customize the Corner Radius?
Here are some reasons why you might want to customize the corner radius of a sheet:
- Design Aesthetics: A custom corner radius can enhance the visual appeal of the sheet, making it more engaging for the user.
- User Experience: Rounded corners can make the sheet feel more integrated and less abrupt, improving the overall user experience.
- Branding: To maintain brand consistency, you might want the sheet’s corner radius to match your app’s design guidelines.
Customizing the corner radius of a sheet in SwiftUI is a straightforward task thanks to the .presentationCornerRadius
modifier. This simple yet powerful modifier allows you to easily customize the appearance of your sheets, making them more aligned with your app’s design and enhancing the user experience.