How to Customize Font Size in iOS SwiftUI TextField
In SwiftUI, a TextField is used to gather input from the user, and the customization of its appearance is essential for user experience. One such aspect is the font size.
This blog post will guide you on how to modify the font size in a SwiftUI TextField, making your app look more appealing and in line with the overall design.
Change Font Size in TextField
The process of changing the font size in a SwiftUI TextField is straightforward and can be achieved using the font
modifier. Below is a code snippet demonstrating how to set the font size of a TextField:
struct ContentView: View {
@State private var text = ""
var body: some View {
TextField("Enter text", text: $text)
.font(.system(size: 20))
.padding()
}
}
@State
Property Wrapper
The @State
property wrapper is used to manage the text entered by the user. It triggers the view to re-render when changes occur.
TextField
The TextField is created with a placeholder "Enter text"
and is bound to the text
state variable.
font
Modifier
The font
modifier is where the magic happens. It’s used to define the font size, in this example set to 20. The .system(size: 20)
applies a system font with the specified size.
padding
Modifier
Padding is added to provide some space around the TextField, ensuring a pleasant layout.
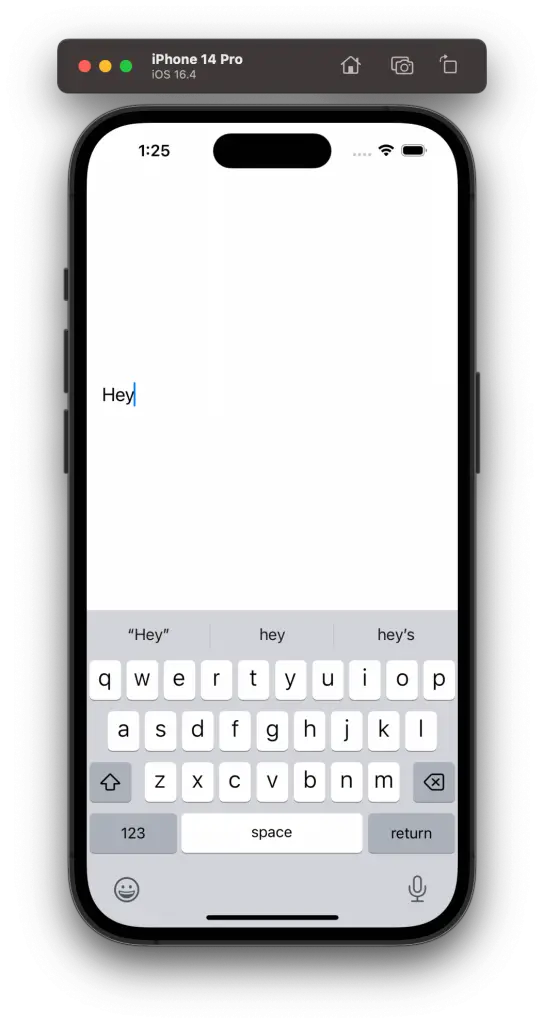
Advanced Customization
Beyond simple font size customization, SwiftUI also allows you to control other font attributes such as weight and design. Here’s an example:
TextField("Enter text", text: $text)
.font(.system(size: 20, weight: .bold, design: .rounded))
This code sets the font size to 20, the weight to bold, and applies a rounded design to the text.
The ability to control font size in a TextField is crucial in crafting an app’s visual identity. SwiftUI’s font
modifier makes this task simple, giving you the power to align text input with your app’s overall design theme.
Experimenting with various font sizes and styles can help you create unique and engaging user interfaces that resonate with your audience.