How to Use Phone Keyboard with iOS SwiftUI TextField
When designing forms that require users to enter a phone number, using the appropriate keyboard type enhances user experience. In SwiftUI, you can specifically request a phone keyboard for a text field.
This guide will walk you through how to set up a text field with a phone keyboard in SwiftUI.
Set up a TextField with Phone Keyboard
SwiftUI provides various keyboard types to suit different inputs, and for phone numbers, you can use the .phonePad
keyboard type. Here’s how you can set up a TextField for phone number input:
struct ContentView: View {
@State private var phoneNumber = ""
var body: some View {
TextField("Enter Phone Number", text: $phoneNumber)
.keyboardType(.phonePad)
.padding()
}
}
Explaination
@State
Property Wrapper
The @State
property wrapper is used to create a variable that will hold the phone number. This ensures that the view updates as the user types.
TextField with Placeholder
A TextField is set up with a placeholder “Enter Phone Number,” guiding users on what to input.
keyboardType
Modifier
The keyboardType
modifier specifies the type of keyboard to display. By setting it to .phonePad
, it ensures that only numbers and the special characters commonly used in phone numbers are available to the user.
Padding
Padding is added around the TextField to give it some breathing room within the layout.
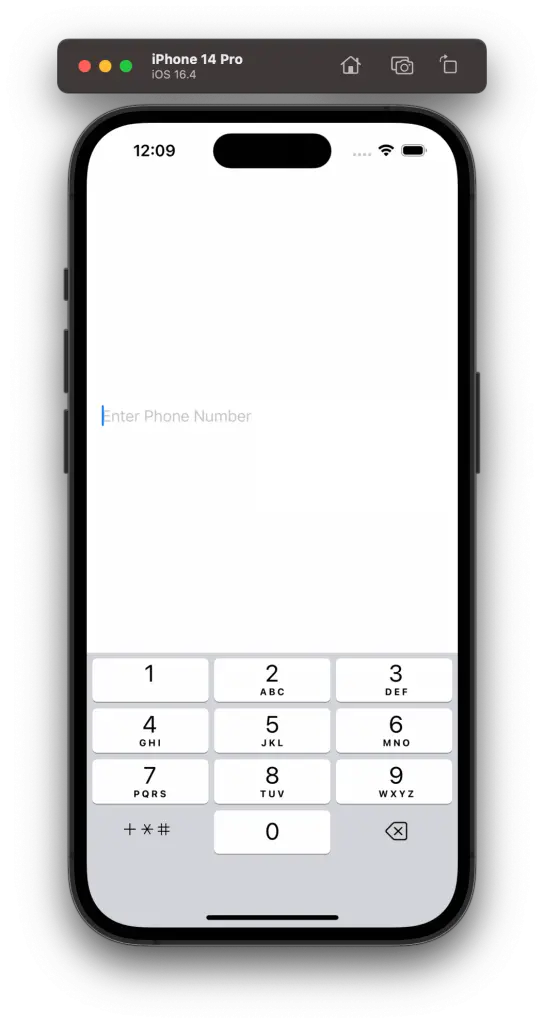
Why Use Phone Keyboard?
Utilizing the phone keyboard in SwiftUI offers several advantages:
- User-Friendly: It helps users input phone numbers quickly and accurately by providing only the necessary keys.
- Consistency: It ensures that the input method aligns with the expected data, creating a consistent user experience.
- Validation: By limiting the keyboard to numbers and specific characters, it makes the validation of phone numbers easier.
Setting up a TextField with a phone keyboard in SwiftUI is simple yet essential when dealing with phone number inputs. By selecting the appropriate keyboard type, you create a more intuitive and efficient experience for users.
Whether for user registration, contact forms, or profile updates, using the phone keyboard can greatly enhance the overall usability of your SwiftUI application.