How to Add LazyVGrid in iOS SwiftUI
SwiftUI, Apple’s innovative and user-friendly framework, has become a popular choice for developing iOS applications. One of the intriguing features of SwiftUI is the LazyVGrid
layout, which creates a flexible and scrollable grid of views.
This post will delve into the details of SwiftUI’s LazyVGrid
using an interactive example different from our base example, but still demonstrating the same principles.
What is LazyVGrid?
The LazyVGrid
is a SwiftUI view that arranges its child views in a grid that grows vertically. It’s “lazy” because it only renders the views that fit on the screen, making it super efficient for handling large collections of views.
How Does LazyVGrid Work?
LazyVGrid
takes two primary parameters:
- An array of
GridItem
objects that define the grid’s columns. - The views to be placed inside the grid.
What is GridItem
The GridItem
struct represents the specifications for a grid column. It accepts three parameters:
size
– Defines the size of the items.spacing
– Determines the space between each grid item.alignment
– Aligns the items along the horizontal axis.
Exploring an Example
Let’s explore a example of LazyVGrid
by creating a grid of colors.
struct ContentView: View {
let columns = [
GridItem(.flexible()),
GridItem(.flexible()),
]
let colors: [Color] = [.red, .green, .blue, .orange, .yellow, .pink, .purple, .gray]
var body: some View {
ScrollView {
LazyVGrid(columns: columns, spacing: 20) {
ForEach(colors, id: \.self) { color in
Rectangle()
.fill(color)
.frame(height: 250)
.cornerRadius(10)
}
}
.padding(.horizontal)
}
}
}
In this example, we’ve defined two columns using GridItem(.flexible())
. This ensures that our grid columns share the available space evenly.
In this ContentView
structure, we define an array of GridItem
and an array of Color
. The ScrollView
wraps our LazyVGrid
which houses our ForEach
loop. This loop cycles through the colors, using each to fill a Rectangle
shape.
Each rectangle has a height of 250 and rounded corners, courtesy of .cornerRadius(10)
. Lastly, padding is applied horizontally to the LazyVGrid
for a more pleasant visual layout.
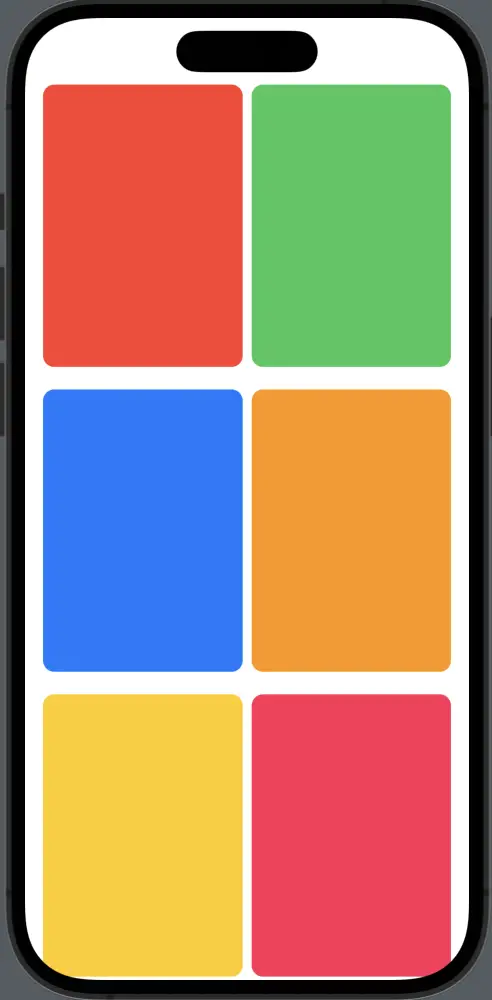
LazyVGrid
is a powerful and efficient tool in SwiftUI that lets you create flexible, scrollable grid interfaces with ease. Understanding and mastering it will significantly enhance your ability to create complex and attractive UIs in SwiftUI.
One Comment