How to Manage LazyVGrid Spacing in iOS SwiftUI
The SwiftUI framework introduced by Apple has transformed how we approach UI development for iOS. Among its myriad of features, SwiftUI’s LazyVGrid
stands out, offering a dynamic way to create vertically oriented grids.
A key aspect of this layout is spacing, which we’ll explore in-depth in this blog post with a colorful example.
LazyVGrid Overview
LazyVGrid
is a SwiftUI view that organizes its children in a grid with vertical growth. The ‘lazy’ part of its name comes from the fact that it only instantiates views as they become visible, thereby saving memory and improving performance.
Role of Spacing in LazyVGrid
Spacing is one of the important aspects in LazyVGrid
. It is set inside the GridItem
object and regulates the distance between items in the grid. This spacing can significantly influence the overall aesthetic and readability of your grid.
LazyVGrid Spacing Example
Let’s now delve into an example that explores the spacing property of LazyVGrid
.
struct ContentView: View {
let columns = [
GridItem(.flexible(), spacing: 20),
GridItem(.flexible(), spacing: 20),
]
let colors: [Color] = [.red, .green, .blue, .orange, .yellow, .pink, .purple, .gray]
var body: some View {
ScrollView {
LazyVGrid(columns: columns, spacing: 40) {
ForEach(colors, id: \.self) { color in
Rectangle()
.fill(color)
.frame(height: 250)
.cornerRadius(10)
}
}
.padding(.horizontal)
}
}
}
In this code, we set the spacing in two places. First, within the GridItem
instances, we set a spacing of 20. This will ensure that there is a gap of 20 points between rows.
Second, we provide a spacing of 40 to the LazyVGrid
. This defines the spacing between the columns. If you run this code, you’ll see a pleasant grid of colored rectangles, each spaced appropriately to provide a clean and visually pleasing layout.
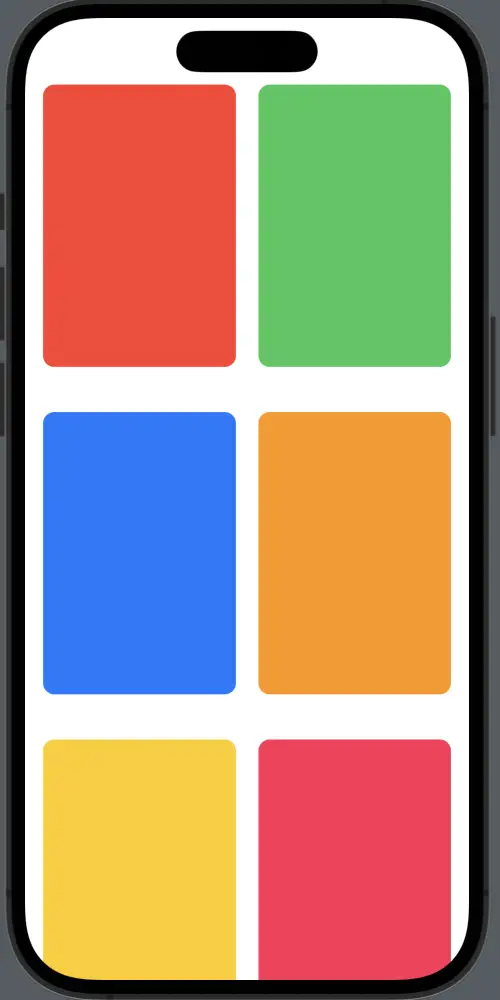
Controlling spacing in SwiftUI’s LazyVGrid
offers a significant advantage when it comes to designing aesthetically pleasing and easily readable grids. Mastering this aspect will take your SwiftUI skills to the next level.