How to Create Simple Grid Layout in iOS SwiftUI
SwiftUI, a powerful tool by Apple, is a paradise for iOS developers. It makes user interface design swift and fun. In this blog post, we will take an in-depth look at one of its components – the grid layout. We will see how to harness the SwiftUI grid layout to create aesthetic and responsive designs.
What is a Grid Layout?
In any design framework, the grid layout holds a significant role. It arranges components in a flexible, systematic structure – like a grid. It helps to create a balanced design that looks good on any screen size.
How to Add Grid Layout in SwiftUI
The code snippet above demonstrates the basic use of grid layout in SwiftUI.
Grid {
GridRow {
Text("Hello")
Image(systemName: "globe")
}
GridRow {
Image(systemName: "hand.wave")
Text("World")
}
}
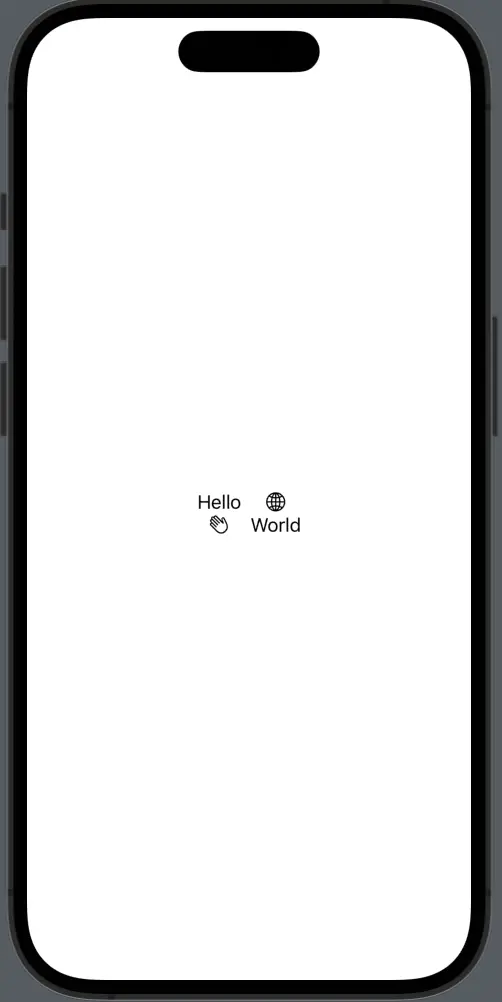
In SwiftUI, GridRow acts as a container for your components. It can hold multiple elements like text, images, buttons, etc. It defines a row inside the grid. Here’s a breakdown of the code above:
GridRow {
Text("Hello")
Image(systemName: "globe")
}
The GridRow has two components: a text saying “Hello” and an image of a globe.
Using the Divider
The Divider is a SwiftUI element that separates sections visually. It’s like an horizontal rule (<hr>
) in HTML. Here’s how it looks in our code:
Divider()
It divides different GridRows, making our UI more readable and structured.
Grid {
GridRow {
Text("Hello")
Image(systemName: "globe")
}
Divider()
GridRow {
Image(systemName: "hand.wave")
Text("World")
}
}
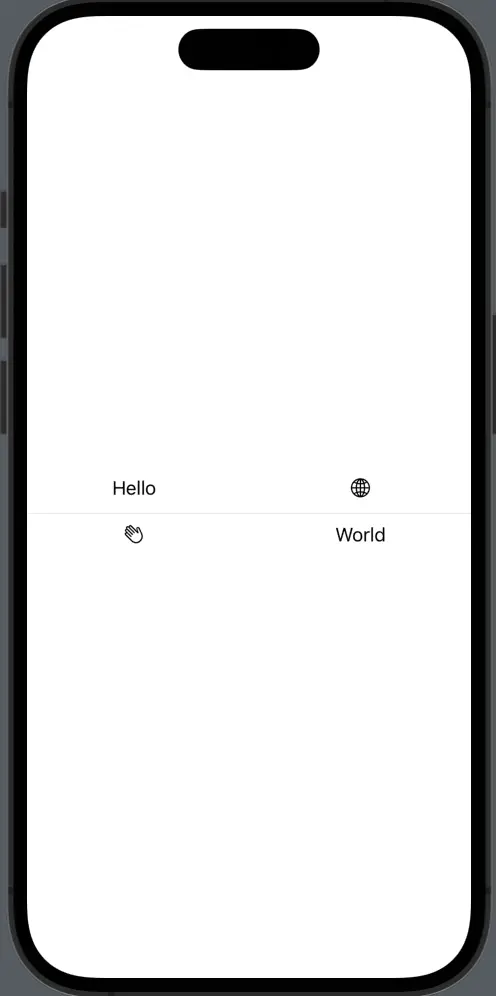
Use the Divider as given below to prevent view from taking up the space.
Grid {
GridRow {
Text("Hello")
Image(systemName: "globe")
}
Divider()
.gridCellUnsizedAxes(.horizontal)
GridRow {
Image(systemName: "hand.wave")
Text("World")
}
}
This restores the grid to the width of the text and images.
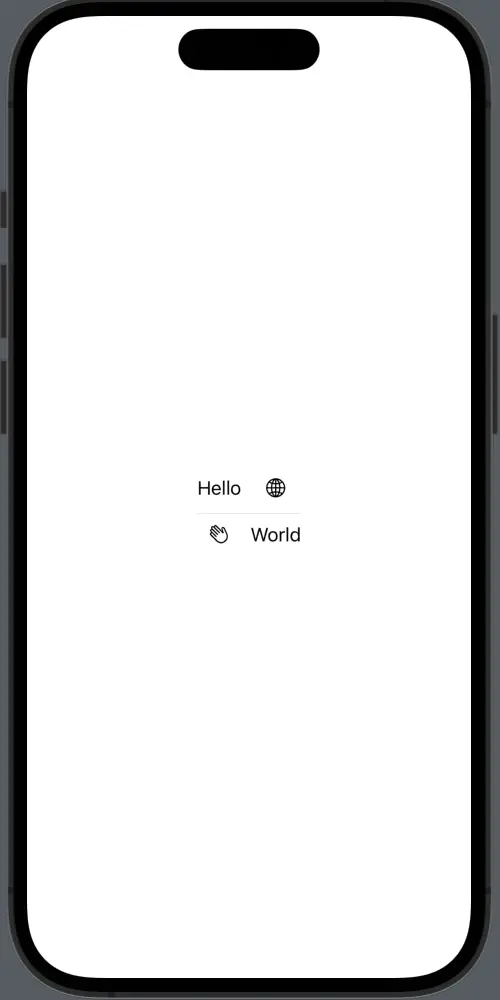
The Bigger Picture
The Grid renders the views immediately. Hence there are chances of performance issue especially when using large data. Prefer LazyVGrid or LazyHGrid for better performance. It’s because they render views only when needed.
The beauty of SwiftUI grid layout is its simplicity and flexibility. This allows you to create complex and beautiful designs with minimal effort.